Authentication: The process or action of verifying the identity of a user or process. Authentication defines whether we have access to certain systems.
Authorization: Authorization is the function of specifying access rights/privileges to resources. Even though we are logged on to a system, authorization defines the access or permission of the user to certain resource or data.
If we have a valid Login ID and Password, we are authenticated with the system. The authorization matrix for the profile defines our authorization to access, create, update, or delete certain data / items in the system.
We know that authorization and custom roles are achieved in our ERP system using PFCG and SU01, In the BTP (ABAP) environment we can achieve it by the following method.
To achieve the custom roles and authorization, we need:
- Authorization Fields
- Authorization Object
- Identity Access Management App (IAM App)
- Identity Access Management Business Catalogs
- Identity Access Management Business Role Template
- Access Control (DCL)
Let’s create a set of custom role and authorization example through an example:
We have a documents table for maintaining documents log.
we will have 3 type of documents:
- A
- B
- C
We have to create role for 3 type of users :
- Users with Read / Update access to Document Type A
- Users with Read / Update access to Document Type B
- Users with Read / Update access to ALL Document Type
Consider that we have the following set of:
Transaction Table: zdocuments
@EndUserText.label : 'DOCUMENTS'
@AbapCatalog.enhancement.category : #NOT_EXTENSIBLE
@AbapCatalog.tableCategory : #TRANSPARENT
@AbapCatalog.deliveryClass : #A
@AbapCatalog.dataMaintenance : #RESTRICTED
define table zdocuments {
key mandt : abap.clnt not null;
key docid : z_document_id not null; “ Document ID
key doctype : z_document_type not null; “Document Type, maintained in zdoctype
doc_name : z_document_type; “Document Name
doc_createdby : z_created_by; “ Document Created by
doc_approvedby : z_approved_by; “ Document Approved by
}
Document Type Master table: zdoctype
@EndUserText.label : 'DOCUMENT Types'
@AbapCatalog.enhancement.category : #NOT_EXTENSIBLE
@AbapCatalog.tableCategory : #TRANSPARENT
@AbapCatalog.deliveryClass : #A
@AbapCatalog.dataMaintenance : #RESTRICTED
define table zdoctype {
key mandt : abap.clnt not null;
key doctype : z_document_type not null; “ Document Type
description : abap.char(255); “ Description For type
}
Message Class: Z_DOC_MESSAGE
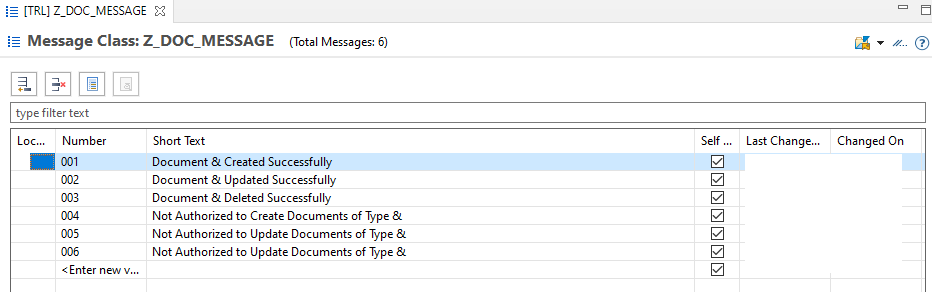
CDS: Z_I_DOCUMENTS
@AbapCatalog.sqlViewName: 'ZDOC'
@AbapCatalog.compiler.compareFilter: true
@AbapCatalog.preserveKey: true
@AccessControl.authorizationCheck: #CHECK
@EndUserText.label: 'Documents'
define root view Z_I_DOCUMENTS
as select from zdocuments
{
key docid as Docid,
key doctype as Doctype,
doc_name as DocName,
doc_createdby as DocCreatedby,
doc_approvedby as DocApprovedby
}
Behavior Definition: Z_I_DOCUMENTS (Here I am Using an Unmanaged Scenario)
unmanaged implementation in class zcl_i_documents unique;
define behavior for Z_I_DOCUMENTS
{
create;
update;
delete;
}
Behavior Implementation: zcl_i_documents
1. Global Class:
CLASS zcl_i_documents DEFINITION
PUBLIC
FINAL for behavior of z_i_documents.
PUBLIC SECTION.
PROTECTED SECTION.
PRIVATE SECTION.
ENDCLASS.
CLASS zcl_i_documents IMPLEMENTATION.
ENDCLASS.
2. Local Types:
CLASS lcl_buffer DEFINITION.
PUBLIC SECTION.
TYPES : BEGIN OF doc_buffer.
INCLUDE TYPE zdocuments AS lv_documents.
TYPES : flag TYPE c LENGTH 1,
END OF doc_buffer.
CLASS-DATA it_documents TYPE TABLE OF doc_buffer.
ENDCLASS.
CLASS lcl_buffer IMPLEMENTATION.
ENDCLASS.
CLASS lcl_handler DEFINITION INHERITING FROM cl_abap_behavior_handler.
PRIVATE SECTION.
METHODS:
create_doc FOR MODIFY
IMPORTING doc_create FOR CREATE z_i_documents,
update_doc FOR MODIFY
IMPORTING doc_update FOR UPDATE z_i_documents,
delete_doc FOR MODIFY
IMPORTING doc_delete FOR DELETE z_i_documents.
ENDCLASS.
CLASS lcl_handler IMPLEMENTATION.
METHOD create_doc.
LOOP AT doc_create INTO DATA(ls_create).
INSERT VALUE #( flag = 'C' lv_documents = CORRESPONDING #( ls_create-%data ) ) INTO TABLE lcl_buffer=>it_documents.
ENDLOOP.
ENDMETHOD.
METHOD update_doc.
IF doc_update IS NOT INITIAL.
LOOP AT doc_update INTO DATA(ls_update).
SELECT SINGLE * FROM zdocuments WHERE docid = @ls_update-Docid AND doctype = @ls_update-Doctype INTO @DATA(ls_db).
INSERT VALUE #( flag = 'U' lv_documents = ls_db ) INTO TABLE lcl_buffer=>it_documents ASSIGNING FIELD-SYMBOL(<ls_buffer>).
IF ls_update-%control-DocName IS NOT INITIAL.
<ls_buffer>-doc_name = ls_update-DocName.
ENDIF.
IF ls_update-%control-DocCreatedby IS NOT INITIAL.
<ls_buffer>-doc_createdby = ls_update-DocCreatedby.
ENDIF.
IF ls_update-%control-DocApprovedby IS NOT INITIAL.
<ls_buffer>-doc_approvedby = ls_update-DocApprovedby.
ENDIF.
ENDLOOP.
ENDIF.
ENDMETHOD.
METHOD delete_doc.
LOOP AT doc_delete INTO DATA(ls_delete).
INSERT VALUE #( flag = 'D' docid = ls_delete-Docid doctype = ls_delete-Doctype ) INTO TABLE lcl_buffer=>it_documents.
ENDLOOP.
ENDMETHOD.
ENDCLASS.
CLASS lcl_saver DEFINITION INHERITING FROM cl_abap_behavior_saver.
PROTECTED SECTION.
METHODS finalize REDEFINITION.
METHODS check_before_save REDEFINITION.
METHODS save REDEFINITION.
ENDCLASS.
CLASS lcl_saver IMPLEMENTATION.
METHOD finalize.
ENDMETHOD.
METHOD check_before_save.
ENDMETHOD.
METHOD save.
DATA lt_documents TYPE TABLE OF zdocuments.
lt_documents = VALUE #( FOR row IN lcl_buffer=>it_documents WHERE ( flag = 'C' ) ( row-lv_documents ) ).
IF lt_documents IS NOT INITIAL.
INSERT zdocuments FROM TABLE @lt_documents.
ENDIF.
lt_documents = VALUE #( FOR row IN lcl_buffer=>it_documents WHERE ( flag = 'U' ) ( row-lv_documents ) ).
IF lt_documents IS NOT INITIAL.
UPDATE zdocuments FROM TABLE @lt_documents.
ENDIF.
lt_documents = VALUE #( FOR row IN lcl_buffer=>it_documents WHERE ( flag = 'D' ) ( row-lv_documents ) ).
IF lt_documents IS NOT INITIAL.
DELETE zdocuments FROM TABLE @lt_documents.
ENDIF.
ENDMETHOD.
ENDCLASS.
Service Definition: Z_SERVDEF_DOC
@EndUserText.label: 'DOCUMENTS'
define service Z_SERVDEF_DOC {
expose Z_I_DOCUMENTS;
}
Service Binding: Z_SERV_DOC
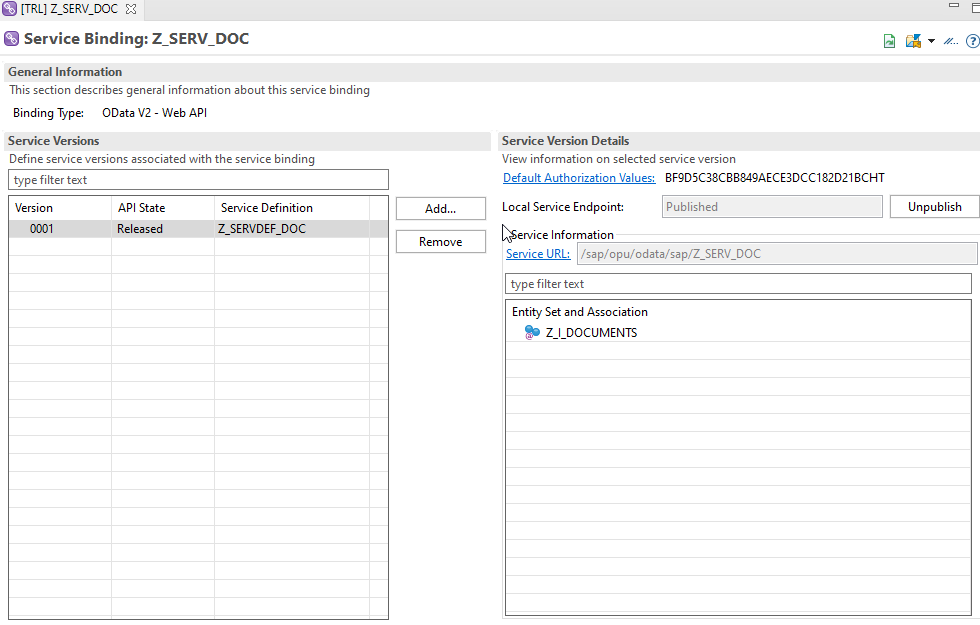
We now need to set up a custom roles for accessing and updating of data from the table zdocuments For each type of document.
Actual Problem Statement
When the user logs in and access the data via our app, it should be automatically sorted and fetched according to the type of user logged in. i.e., If a user with Read / Update permission for document type B logs in, only documents with type B should be shown to him. If he has permission to create documents, he should be only able to create documents of type B. If he has the read permission for all documents, he should only be allowed to update Documents of type B.
How are we going to achieve this?
When we create users (Business Users ) in our Instance via cockpit, we have seen that we should assign User Roles for the user to be a part of the system. Some of the Predefined roles we see are : BR_DEVELOPER, BR_ADMIN etc, All the users in the system don’t need all these access permissions. As mentioned in the problem statement above, we might need users with limited Read/Update/Create/Delete functionalities.
For this We need to create roles, which will be listed in the Roles section in the cockpit.
We can use the Catalogue / Catalogues mentioned in the cockpit to create Roles
Or
We can create Role templates from the backend system and use those to create roles.
- For Creating role templates, we need IAM Catalogs.
- For IAM Catalogs we need IAM APPS.
- For IAM Apps we need Authorization Objects.
- For Authorization Objects we need Authorization Fields.
Inside the IAM Apps we will be mentioning our Service Binding name, Authorization objects and the Values for Authorization Fields. - The IAM Apps are used:
- Access Controls (Data Control Language): At the time of entity read (CDS via Service), if the CDS has an access control then the rows which satisfy the access control are fetched and send via Service.
- AUTHORITY-CHECK OBJECT (Usually inside Behavior Implementations): At the time Create / Update / Delete we will pass the authorization values to this object to check whether the user is authorized to perform the action. If the AUTHORITY-CHECK OBJECT return sy-subrc as 0 with the values passed, then the user has permission
- Access Controls (Data Control Language): At the time of entity read (CDS via Service), if the CDS has an access control then the rows which satisfy the access control are fetched and send via Service.
Now we will see how the above mentioned objects are created and which values are maintained in IAM APPS for different Users:
Authorization Fields (SU20): The authorization fields are in the form of single values or range value and this value sets are known as authorizations. You can allow all the values or empty field as a permissible value and system checks these authorization value sets.
Creation: Right Click on the package -> New ->Other ABAP Repository Object
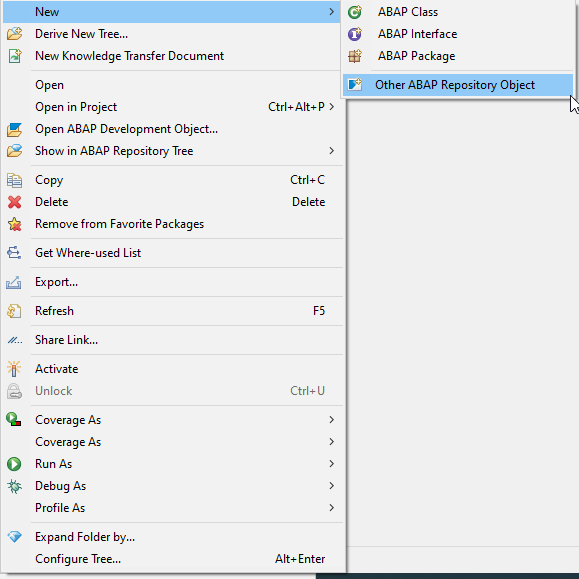
Choose Authorization -> Authorization Field
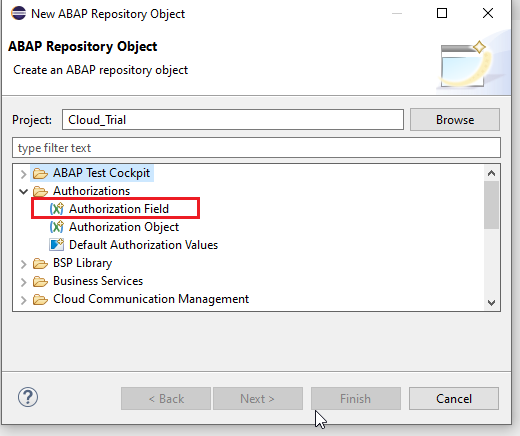
Name the object -> Next -> Select a Transport Request -> Finish. We will Name our Object Z_DOC
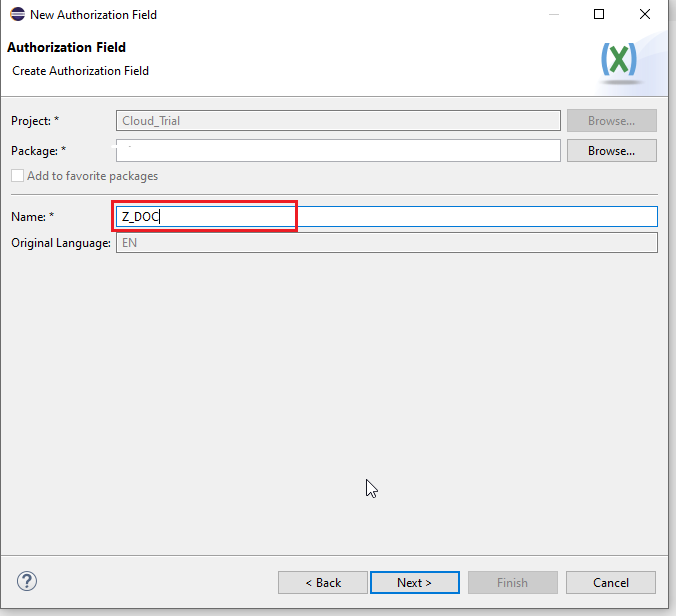
Enter the name of data element which we are going to use and Check Table. Activate the Authorization Field.
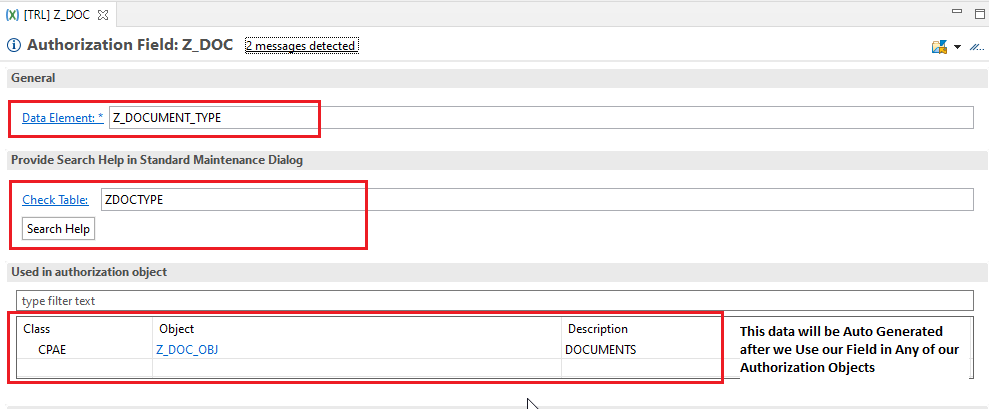
- Data Element: Data element should have an underlying domain, else on activating the authorization field will return error.
- Check Table: Master table where the values for the authorization field are maintained which could be used as search help.
Authorization Objects (SU21): An authorization object consists of up to 10 authorization fields. Combinations of authorization fields, which represent data and activities, are used to grant and check authorizations. Authorization objects are grouped together in authorization object classes
Creation: Right Click on the package -> New ->Other ABAP Repository Object
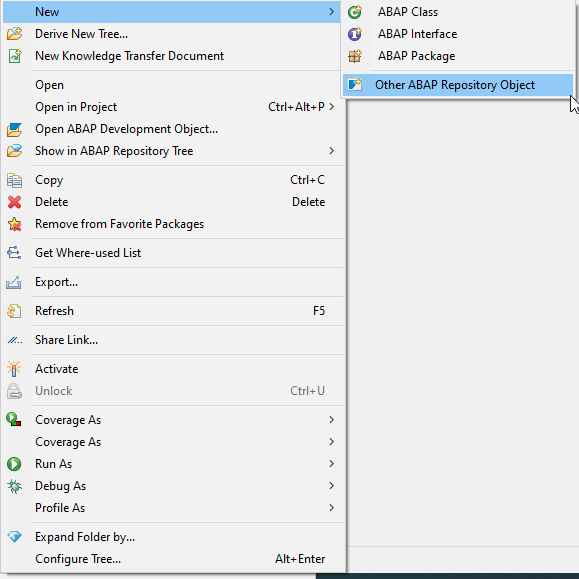
Choose Authorization -> Authorization Object
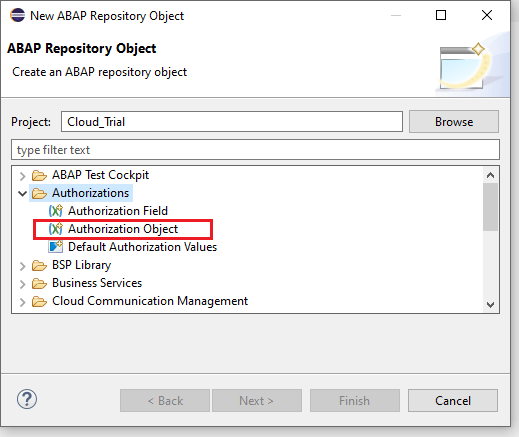
Name the object, Description -> Next -> Select a Transport Request -> Finish. We will Name our Object Z_DOC_OBJ
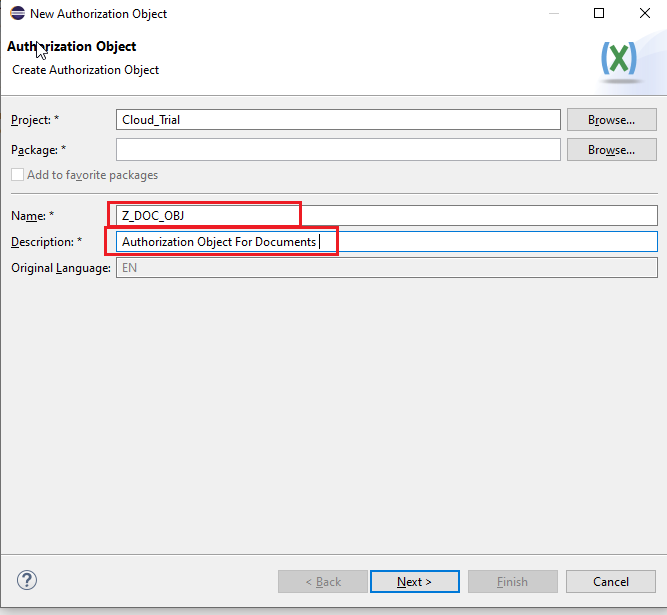
Enter our authorization field / fields, actvt is an sap standard and all our objects will have this field. Values for actvt will be mentioned in the permitted activities tab. Activate the Authorization Object.
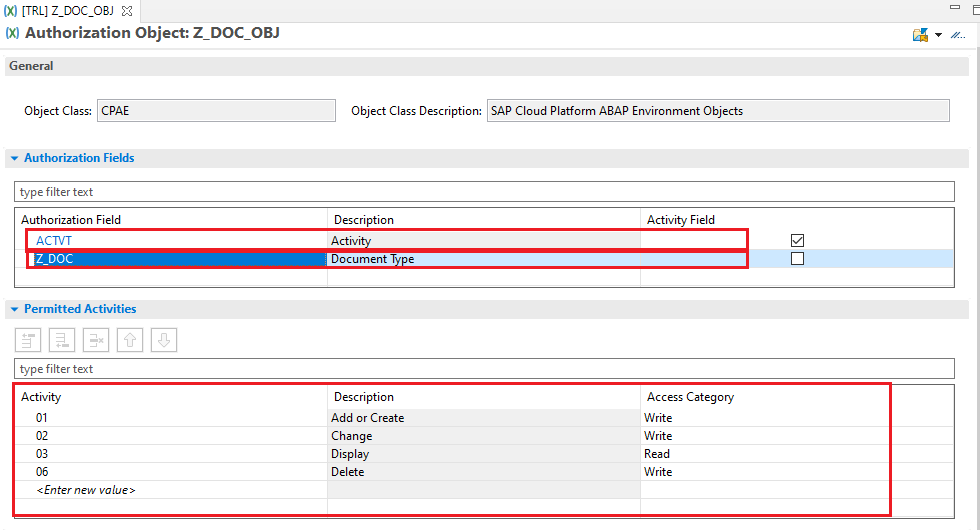
Identity Access Management App (IAM App): IAM Apps Consist of one or more authorization Objects, the value for authorization fields in the authorization objects are mentioned here. According to those values maintained here, the actual authorization matrix works. not only the authorization objects and field values are maintained but also services are also maintained here, the authorization will only act on the services mentioned in the IAM APP.
Creation: Right Click on the package -> New ->Other ABAP Repository Object
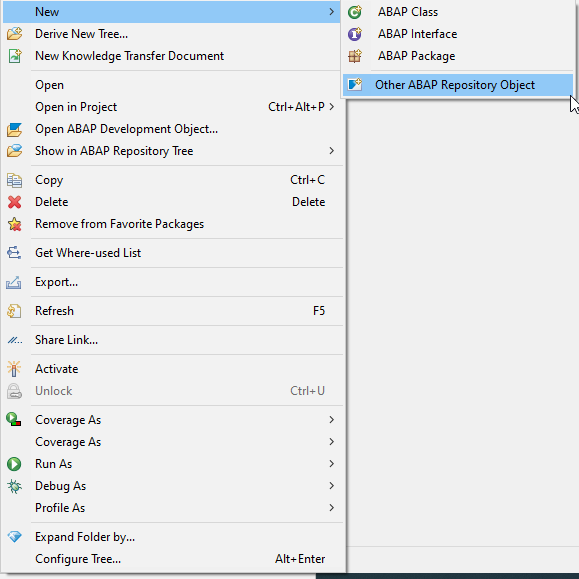
Choose cloud identity and access management -> IAM App
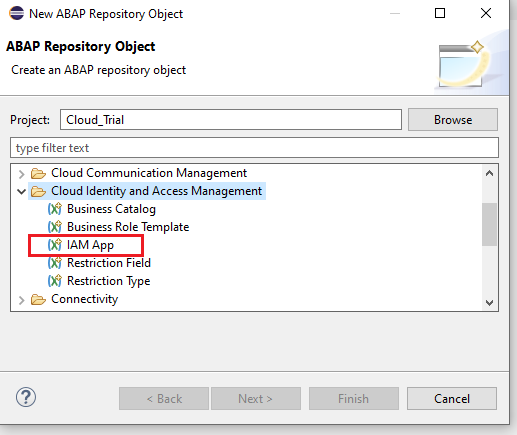
Name the object, Description -> Next -> Select a Transport Request -> Finish. We will Name our Object ZDOC_TYPEA_EXT
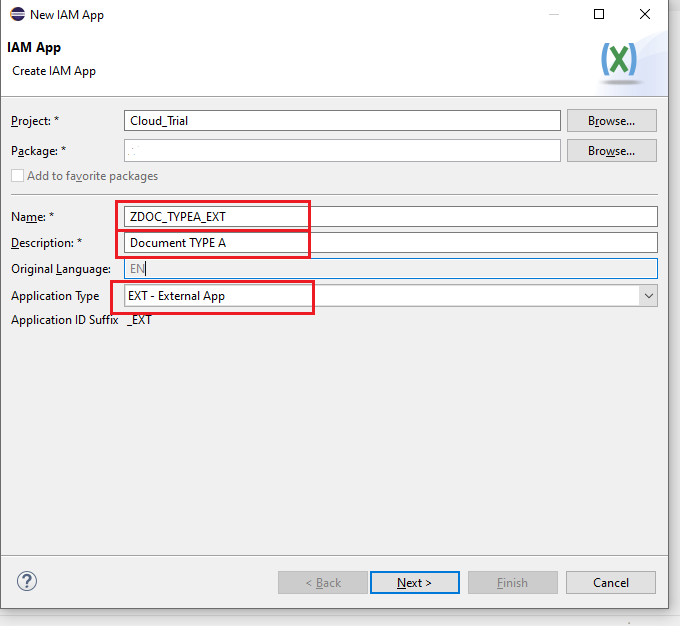
- Navigate to Services tab
- Using the insert button, we will add our already created service.
- Since our service is ODATA V2 we will choose it from the Drop Down
- We will give the service binding name
Note : Authorization will only work on the entities mentioned in the service binding, User will only get data from the entities mentioned in the binding
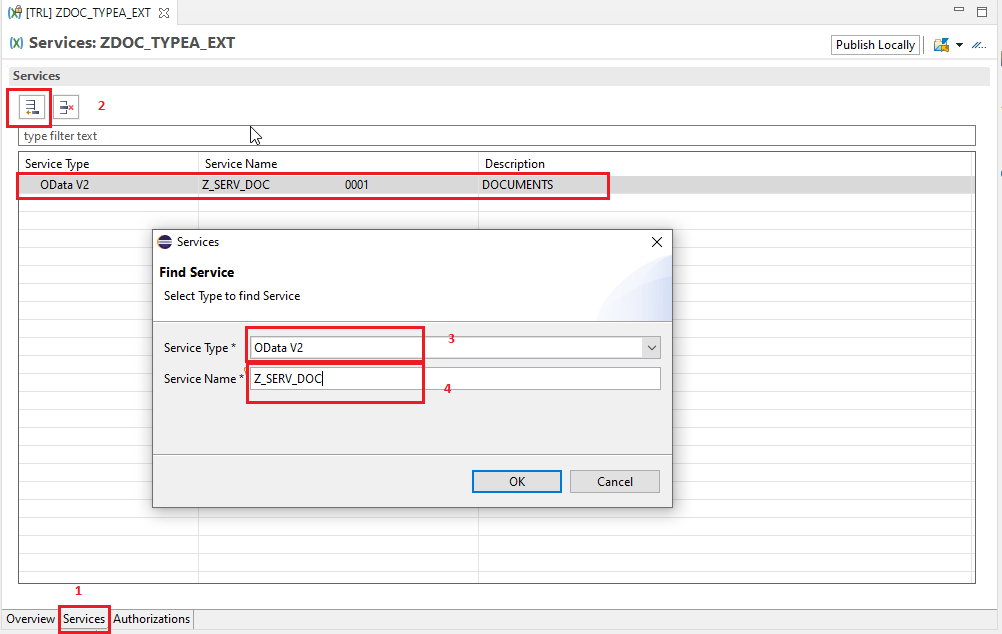
- Navigate to the Authorizations tab
- Using the insert button we will add our already created Authorization object
- We will enter the name of our authorization object
- Authorization fields in the objects will be listed
- Authorization object will be added to the IAM App
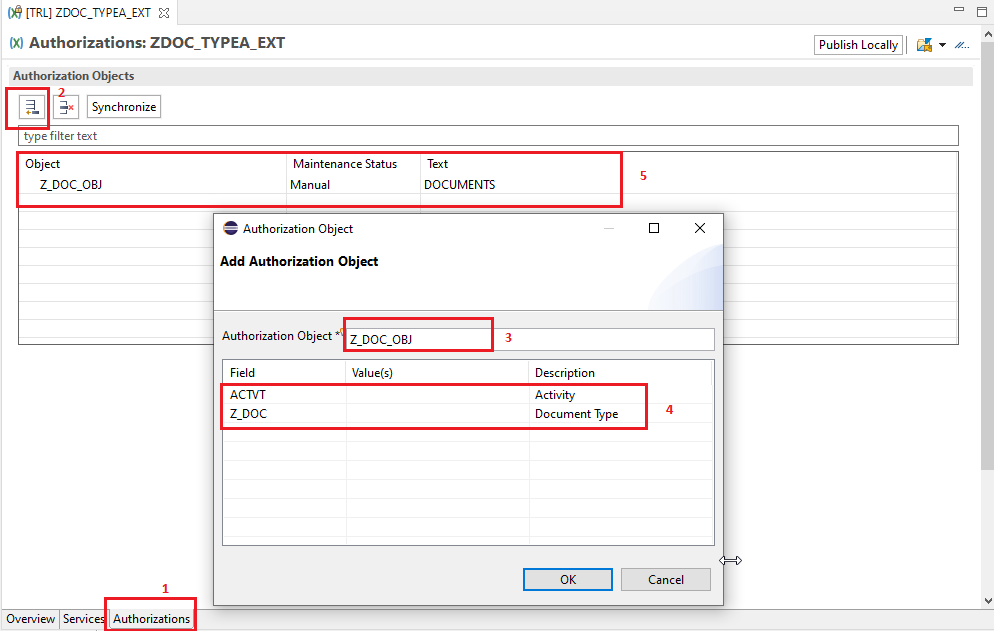
- Click on the Authorization object name
- List of fields will be displayed on the bottom, from the list click on each field to insert desired data
- Enter the data / range of values to be checked with the table field, for actvt check all the boxes in the value area.
- Since we are creating our IAM APP for the users who can access and edit type A documents, this value will be maintained the field value list.
- Activate the object
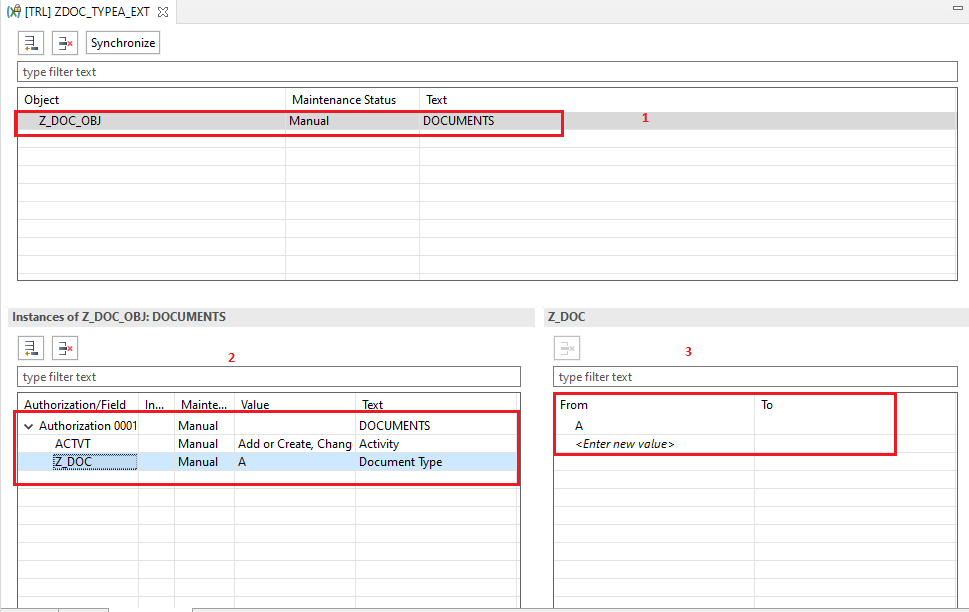
Similarly we will create IAM APPS for TYPE B and for All documents with the following name
ZDOC_TYPEB_EXT:
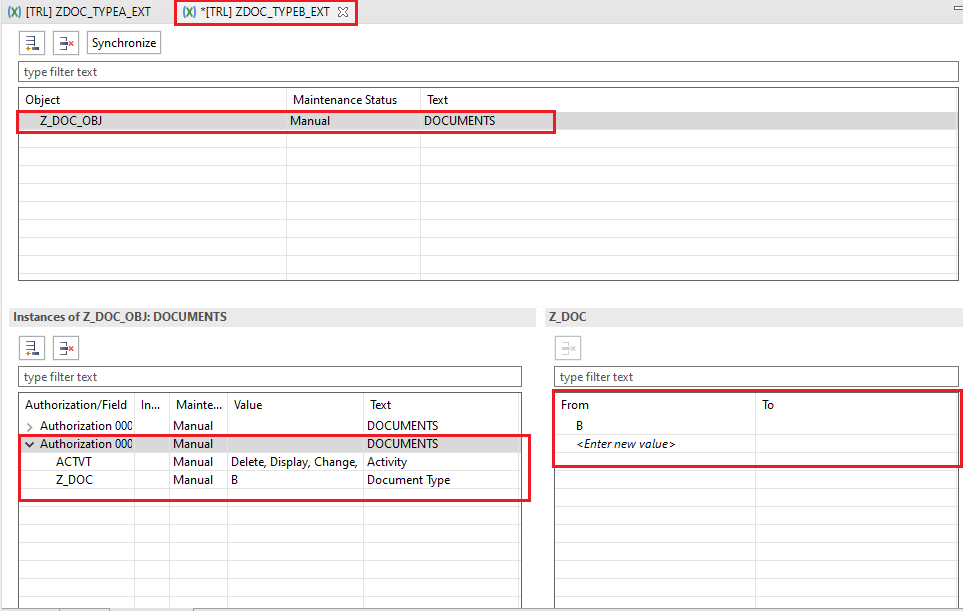
ZDOC_TYPE_ALL_EXT : If we want all values to be passed we could use * in the value area (* is a wildcard character indicating “for all “)
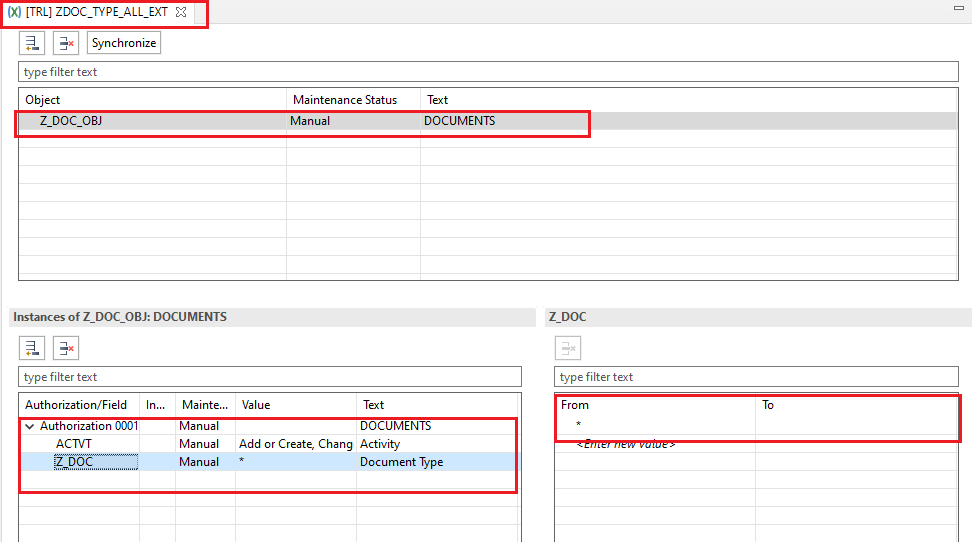
Identity Access Management Business Catalogs (IAM Business Catalogs): Business Catalogs consist of one or more IAM APPS. These IAM Catalogs are used to create business roles, We could create a business role using the role template in the backend or we can publish the catalog locally ( pushing it to the cockpit ) and create business roles in the cockpit and use them. Either way works fine and similar but the approach is our choice.
Creation: Right Click on the package -> New ->Other ABAP Repository Object
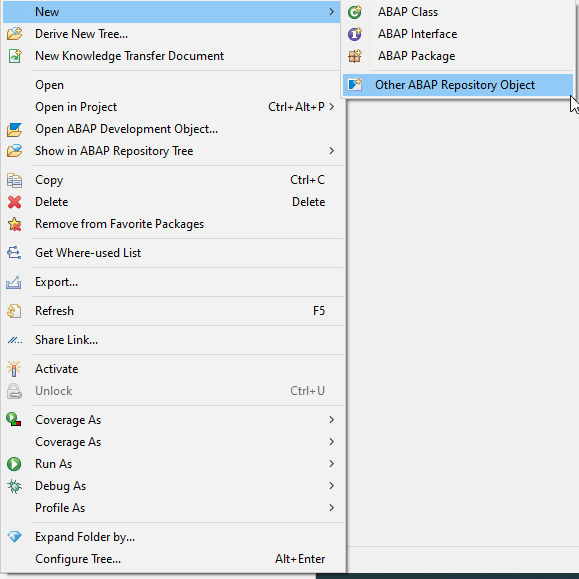
Choose cloud identity and access management -> Business Catalog
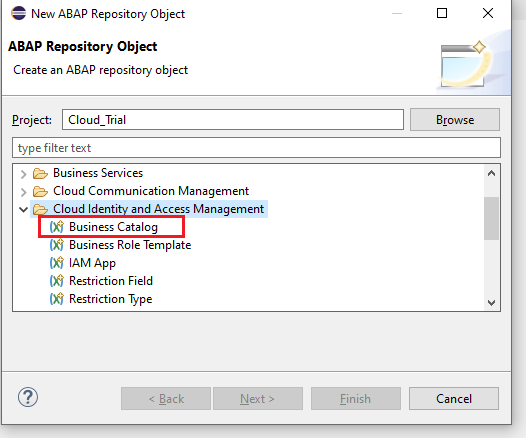
Name the object, Description -> Next -> Select a Transport Request -> Finish. We will Name our Object Z_DOC_TYPEA
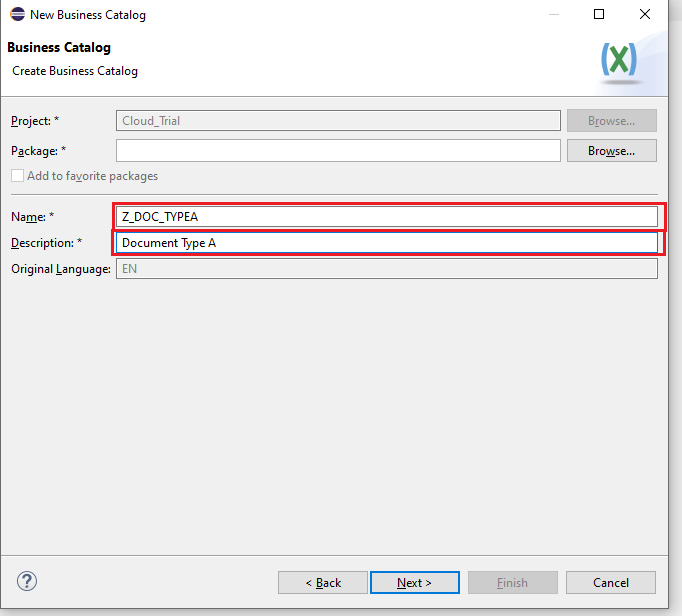
- Navigate to apps tab
- Click on the add button
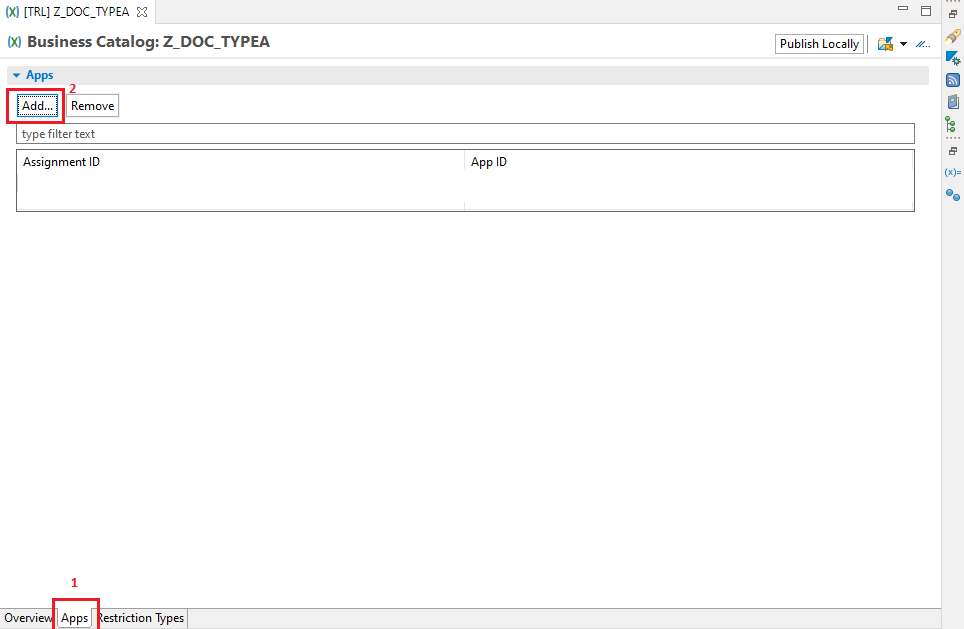
- New window will pop up, which will create the IAM APP to IAM Catalogue Assignment
- Provide name of IAM APP to be used, Name of Assignment, Description -> Next -> Attach it to a TR.
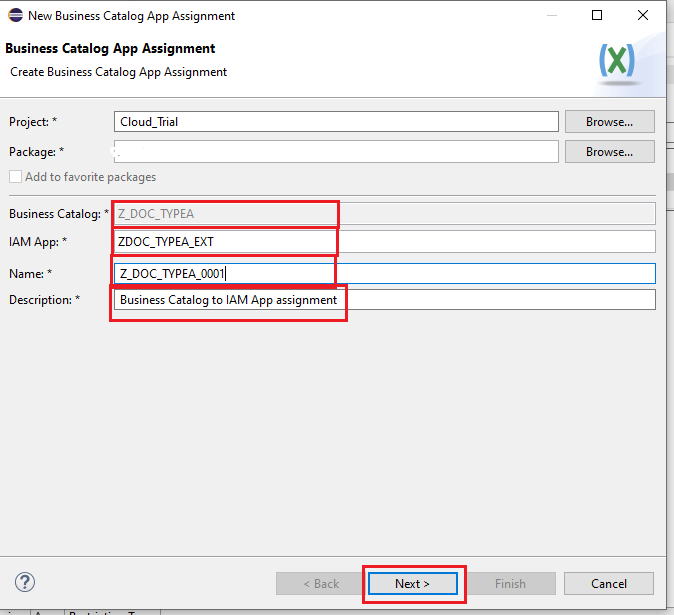
Activate the Business Catalog. If we are going to create the role from cockpit, Press the publish locally button and activate again. Our business catalog will be visible in the business catalogs in cockpit.
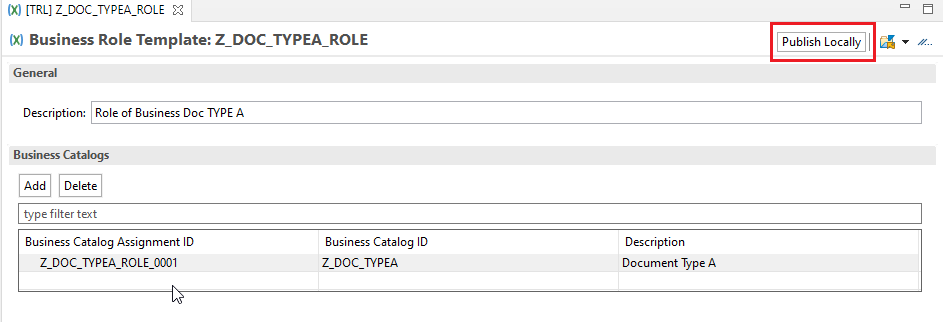
In the previous step, while adding the IAM app we have given the IAM App name and an assignment name in the new pop up window, which will actually create an IAM App to catalog assignment and we can see it in the project structure.
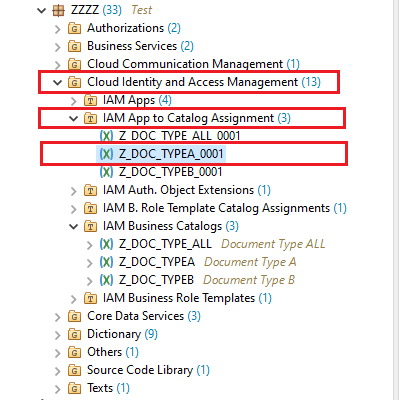
Similarly we will create the Business catalogs for :
- Type B :
- Catalog : Z_DOC_TYPEB
- Assignment : Z_DOC_TYPEB_0001
- Type ALL :
- Catalog : Z_DOC_TYPE_ALL
- Assignment : Z_DOC_TYPE_ALL_0001
Business Role Template: A Business role template provides users with authorization to access / update data in the system. A role template will consist of one or more Business catalogs. Business roles could be created either in the cockpit or in the ABAP system. In our approach the role template will be created in the ABAP system and will be pushed to the cockpit via pushing locally.
Creation: Right Click on the package -> New ->Other ABAP Repository Object
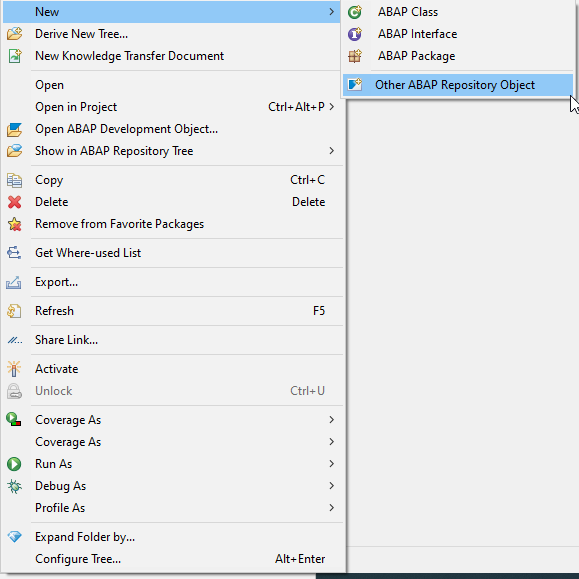
Choose cloud identity and access management -> Business Role Template
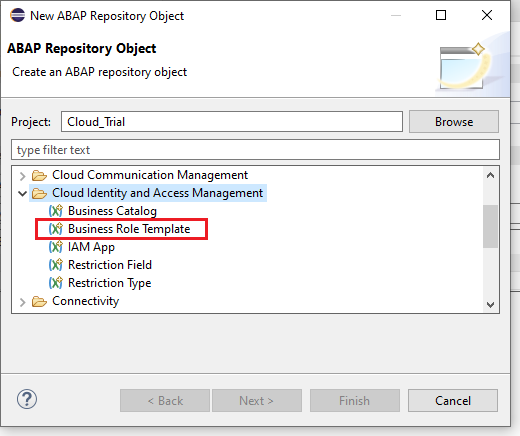
Name the object, Description -> Next -> Select a Transport Request -> Finish. We will name our object : Z_DOC_TYPEA_ROLE.
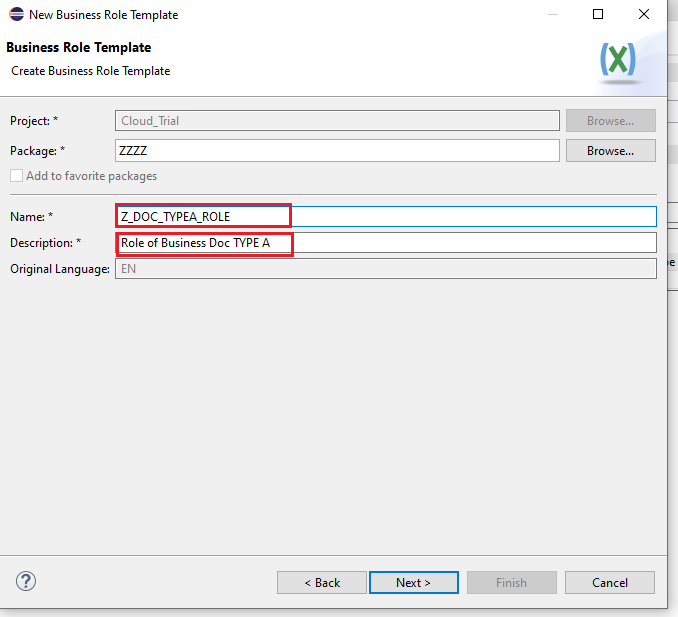
- Click on the add button -> New Window pops up
- Enter the Catalogue Name
- Enter the assignment name
- Next -> Attach it to a TR -> Finish
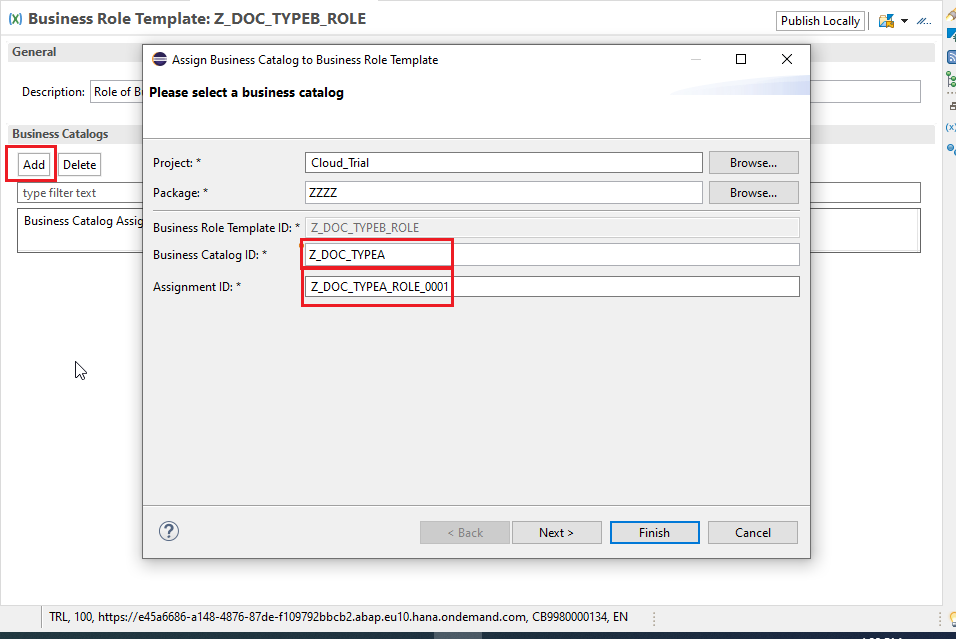
Activate the Role template, Click on the Publish Locally button in order to make the role template visible on our cockpit.
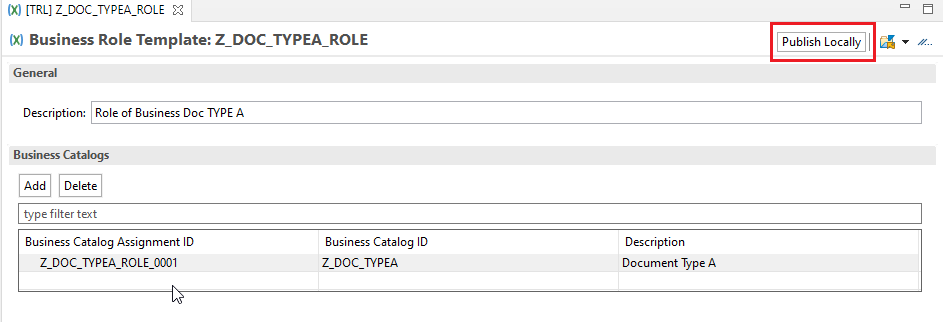
In the previous step, while adding the Catalog we have given the Catalog name and an assignment name in the new pop up window, which will actually create an Catalog to role assignment and we can see it in the project structure.
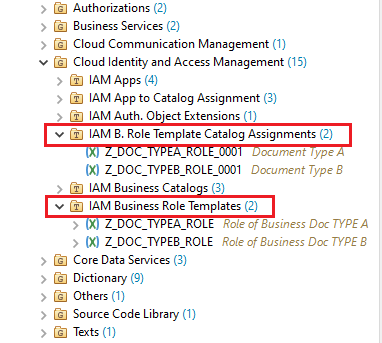
Similarly we will create the Business Roles for :
- Type B :
- Role Template : Z_DOC_TYPEB_ROLE
- Assignment : Z_DOC_TYPEB_ROLE_0001
- Type ALL :
- Role Template : Z_DOC_TYPE_ALL_ROLE
- Assignment : Z_DOC_TYPE_ALL_ROLE_0001
Access Control: These are basically data control language, which restricts the data returned from a CDS entity. In our case we will restrict the data using the IAM App and Authorization Field data maintained in the IAM app. We just basically check whether the values maintained in the IAM APP matches with a specific field in the table. We can also say that it behaves like a dynamic where condition with respect to a table field applied above the CDS view filtering the selection result again.
Creation: Right Click on the package -> New ->Other ABAP Repository Object
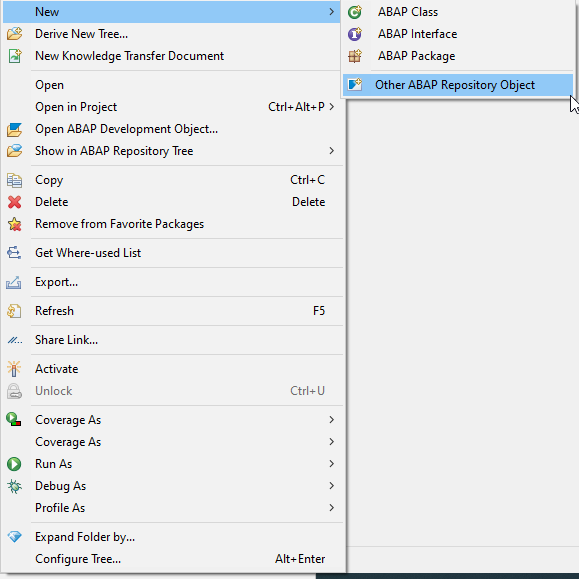
Choose Core Data Services -> Access Controls
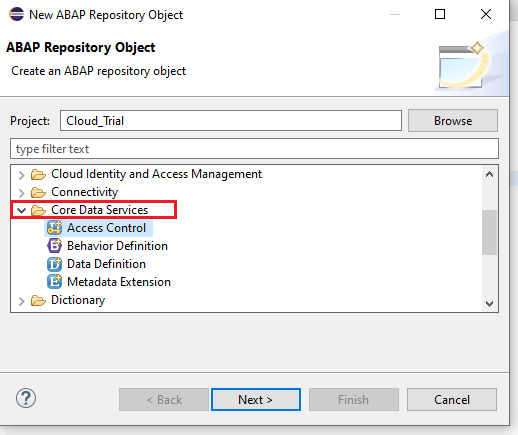
Name the object, Description -> Next -> Select a Transport Request -> Next. We will name our object : ZDOC_FETCH.
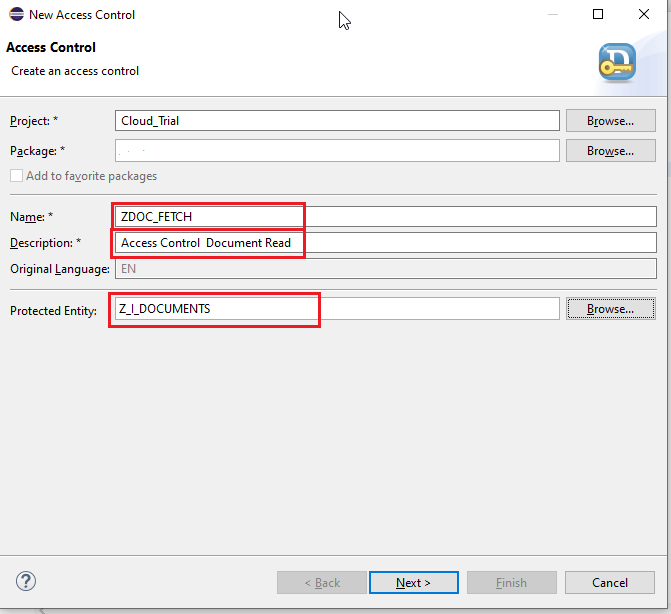
We can choose the Type of access control we want from the list, Here we will be going with the PFCG Concept.
PFCG stands for Perfectly Functionally Co-coordinating Group, we are already familiar with PFCG from the past ( PFCG ). Defines a role that grants instance-specific access to a CDS entity based on authorizations derived from PFCG roles assigned to the current user.
Types of Access Controls:
- Role with Simple condition: Defines a role that grants instance-specific access to a CDS entity through literal conditions or current user name.
- Role with PFCG aspect: Defines a role that grants instance-specific access to a CDS entity based on authorizations derived from PFCG roles assigned to the current user.
- Role with Inherited condition: Inheritance enables you to create a CDS access control for an entity that uses a protected entity as data source without the need to copy the conditions.
- Role with Generic aspect: Defines a role that grants instance-specific access to a CDS entity based on values from a generic aspect defined in a generic aspect definition.
- Generic aspect: Defines how a generic aspect provides the authorized values for the current user based on a CDS entity.
- Role with unrestricted access: Defines a role that grants unrestricted access to a CDS entity.
- Role with privileged access: Defines a role that denies access to a CDS entity unless the access uses a privileged channel. Access may be possible anyway, if granted through other CDS access controls.
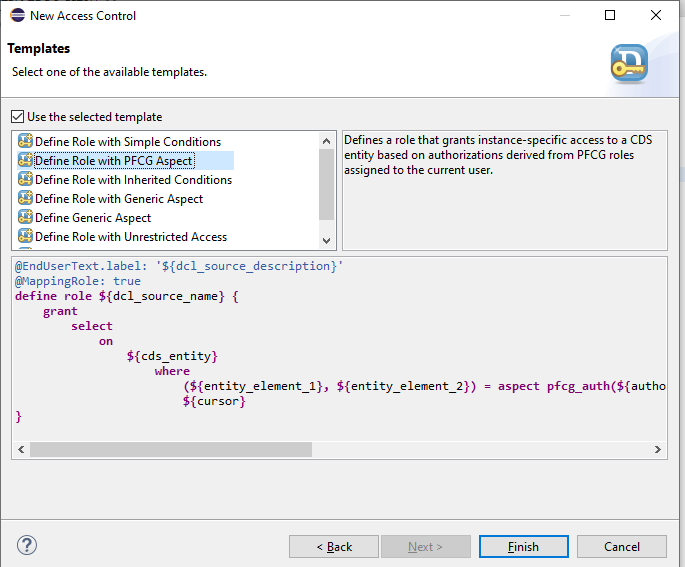
Our Access control should look like:
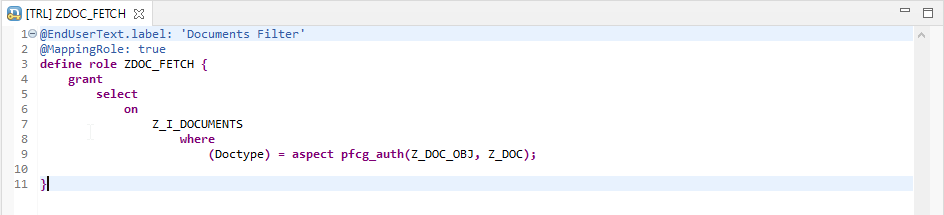
Syntax:
@EndUserText.label: '${dcl_source_description}'
@MappingRole: true
define role ${dcl_source_name} {
grant
select
on
${cds_entity}
where
(${entity_element_1}, ${entity_element_2}) = aspect pfcg_auth(${authorization_object}, ${authorization_field_1}, ${authorization_field_2}, ${filter_field_1} = '${filter_value_1}');
${cursor}
}
Our Code:
@EndUserText.label: 'Documents Filter'
@MappingRole: true
define role ZDOC_FETCH {
grant
select
on
Z_I_DOCUMENTS //Name Of CDS On which our Access Control Should Work on
where
(Doctype) = aspect pfcg_auth(Z_DOC_OBJ, Z_DOC);
//Doctype : Field on the table
//Z_DOC_OBJ : Authorization Object Name
//Z_DOC: Authorization Field in the ob ject
}
How It Works:
whenever the CDS is called for a read, The data retrieved will be filtered based on the doctype field with values mentioned in the Authorization field (Z_DOC) inside the Authorization object (Z_DOC_OBJ) which is used in the IAM APP for the current user role.
In case of a read, the above mentioned scenario happens. What about the case of Create / Update ?
We will have to check the authority using AUTHORITY-CHECK OBJECT. we will implement the changes in the behavior implementation class.
After the code change the our class ( Local Types ) should look like :
CLASS lcl_buffer DEFINITION.
PUBLIC SECTION.
TYPES : BEGIN OF doc_buffer.
INCLUDE TYPE zdocuments AS lv_documents.
TYPES : flag TYPE c LENGTH 1,
END OF doc_buffer.
CLASS-DATA it_documents TYPE TABLE OF doc_buffer.
ENDCLASS.
CLASS lcl_buffer IMPLEMENTATION.
ENDCLASS.
CLASS lcl_handler DEFINITION INHERITING FROM cl_abap_behavior_handler.
PRIVATE SECTION.
METHODS:
create_doc FOR MODIFY
IMPORTING doc_create FOR CREATE z_i_documents,
update_doc FOR MODIFY
IMPORTING doc_update FOR UPDATE z_i_documents,
delete_doc FOR MODIFY
IMPORTING doc_delete FOR DELETE z_i_documents.
ENDCLASS.
CLASS lcl_handler IMPLEMENTATION.
METHOD create_doc.
LOOP AT doc_create INTO DATA(ls_create).
"Authorization Check
AUTHORITY-CHECK OBJECT 'Z_DOC_OBJ' ID 'ACTVT' FIELD '01'
ID 'Z_DOC' FIELD ls_create-Doctype.
IF sy-subrc <> 0.
DATA(item_msg1) = new_message( id = 'Z_doc_message'
number = '004'
severity = cl_abap_behv=>ms-error
v1 = ls_create-Doctype
v2 = ''
v3 = ''
v4 = '').
APPEND VALUE #( %cid = ls_create-%cid docid = ls_create-docid )
TO mapped-z_i_documents.
APPEND VALUE #( %cid = ls_create-%cid
docid = ls_create-docid
%msg = item_msg1 ) TO reported-z_i_documents.
ENDIF.
"Authorization Check - end
INSERT VALUE #( flag = 'C' lv_documents = CORRESPONDING #( ls_create-%data ) ) INTO TABLE lcl_buffer=>it_documents.
ENDLOOP.
ENDMETHOD.
METHOD update_doc.
IF doc_update IS NOT INITIAL.
LOOP AT doc_update INTO DATA(ls_update).
SELECT SINGLE * FROM zdocuments WHERE docid = @ls_update-Docid AND doctype = @ls_update-Doctype INTO @DATA(ls_db).
INSERT VALUE #( flag = 'U' lv_documents = ls_db ) INTO TABLE lcl_buffer=>it_documents ASSIGNING FIELD-SYMBOL(<ls_buffer>).
IF ls_update-%control-DocName IS NOT INITIAL.
<ls_buffer>-doc_name = ls_update-DocName.
ENDIF.
IF ls_update-%control-DocCreatedby IS NOT INITIAL.
<ls_buffer>-doc_createdby = ls_update-DocCreatedby.
ENDIF.
IF ls_update-%control-DocApprovedby IS NOT INITIAL.
<ls_buffer>-doc_approvedby = ls_update-DocApprovedby.
ENDIF.
"Authorization Check
AUTHORITY-CHECK OBJECT 'Z_DOC_OBJ' ID 'ACTVT' FIELD '02'
ID 'Z_DOC' FIELD ls_update-Doctype.
IF sy-subrc <> 0.
DATA(item_msg1) = new_message( id = 'Z_DOC_MESSAGE'
number = '005'
severity = cl_abap_behv=>ms-error
v1 = ls_update-doctype
v2 = ''
v3 = ''
v4 = '').
APPEND VALUE #( %cid = ls_update-%cid_ref docid = ls_update-docid )
TO mapped-z_i_documents.
APPEND VALUE #( %cid = ls_update-%cid_ref
docid = ls_update-docid
%msg = item_msg1 ) TO reported-z_i_documents.
ENDIF.
"Authorization Check-end
ENDLOOP.
ENDIF.
ENDMETHOD.
METHOD delete_doc.
LOOP AT doc_delete INTO DATA(ls_delete).
INSERT VALUE #( flag = 'D' docid = ls_delete-Docid doctype = ls_delete-Doctype ) INTO TABLE lcl_buffer=>it_documents.
ENDLOOP.
ENDMETHOD.
ENDCLASS.
CLASS lcl_saver DEFINITION INHERITING FROM cl_abap_behavior_saver.
PROTECTED SECTION.
METHODS finalize REDEFINITION.
METHODS check_before_save REDEFINITION.
METHODS save REDEFINITION.
ENDCLASS.
CLASS lcl_saver IMPLEMENTATION.
METHOD finalize.
ENDMETHOD.
METHOD check_before_save.
ENDMETHOD.
METHOD save.
DATA lt_documents TYPE TABLE OF zdocuments.
lt_documents = VALUE #( FOR row IN lcl_buffer=>it_documents WHERE ( flag = 'C' ) ( row-lv_documents ) ).
IF lt_documents IS NOT INITIAL.
INSERT zdocuments FROM TABLE @lt_documents.
ENDIF.
lt_documents = VALUE #( FOR row IN lcl_buffer=>it_documents WHERE ( flag = 'U' ) ( row-lv_documents ) ).
IF lt_documents IS NOT INITIAL.
UPDATE zdocuments FROM TABLE @lt_documents.
ENDIF.
lt_documents = VALUE #( FOR row IN lcl_buffer=>it_documents WHERE ( flag = 'D' ) ( row-lv_documents ) ).
IF lt_documents IS NOT INITIAL.
DELETE zdocuments FROM TABLE @lt_documents.
ENDIF.
ENDMETHOD.
ENDCLASS.
If you look at the code, you will notice that I have made a change in methods create_doc and update_doc. I have added an authority-check in both the methods, what this actually does is :
If you look at the code,
AUTHORITY-CHECK OBJECT 'Z_DOC_OBJ' ID 'ACTVT' FIELD '01'
ID 'Z_DOC' FIELD ls_create-Doctype.
Definition: This statement checks whether an authorization is entered in the user master record of the current user or of the user specified in user for the authorization object specified in auth_obj, and whether this authorization is sufficient for the request specified in the statement. If the addition FOR USER is not specified, the authorization of the current user is checked.
How It Works:
I am passing the object name ( Z_DOC_OBJ ) and the Field names ( ACTVT, Z_DOC ) and their values ( ‘01 ‘ and the document type).
As we see in the case of Access controls, the authority-check will use the authorization object, fields mentioned and their respective values to check with the IAM App and Values mentioned in the APP. If the values match statement returns a sy-subrc value of 0.
sy-subrc | Meaning |
0 | Authorization check successful or no check was performed. An authorization for the authorization object was found in the user master record. Its value sets include the specified values. |
4 | Authorization check not successful. One or more authorizations were found for the authorization object in the user master record and they include the value sets, but not the values specified, or incorrect authorization fields or too many fields were specified. |
12 | No authorization was found for the authorization object in the user master record. |
24 | This return code is no longer set. |
40 | An invalid user name was specified in user. |
How does the whole scenario works?
Consider that we have 2 Users in our system
- Vinu (Top level User who has the authorization to Read / Edit / Create all documents)
- Mark (Guest User who has the permission to Read / Edit / Create Documents of type A)
Vinu will be having :
- Role : Z_DOC_TYPE_ALL_ROLE
- Catalog : Z_DOC_TYPE_ALL
- IAM APP : ZDOC_TYPE_ALL_EXT
- Value For Authorization field Z_DOC : *
So when Vinu Logs in to the system and request a data read from the Documents Table via CDS, The access control will act up on the CDS view. Since ‘ * ‘ is maintained for Z_DOC all the documents will be fetched for him (* is wild card character used to denote FOR ALL ).
When the user Vinu Creates or Updates a document, in the behavior implementation we had a check using the AUTHORITY-CHECK, this will also use the value maintained in the Z_DOC field in the IAM App to match with the document type in the table.
Mark will be having :
- Role : Z_DOC_TYPEA_ROLE
- Catalog : Z_DOC_TYPEA
- IAM APP : ZDOC_TYPEA_EXT
- Value For Authorization field Z_DOC : A
When Mark logs in instead of using the ‘*’ for checks ‘A’ Will be used, so only documents of type A will be fetched and Mark will only be able to Create / Edit documents of Type A.
Playing around with authorization and values seem a bit tricky but when you get the working of it, its actually a simple mechanism. Since each level of objects can contain one or more of objects in the previous level (Authorization object can have Multiple fields, IAM App can have multiple Objects and so on), So its pretty much simple to construct any tough level of authorization matrix.