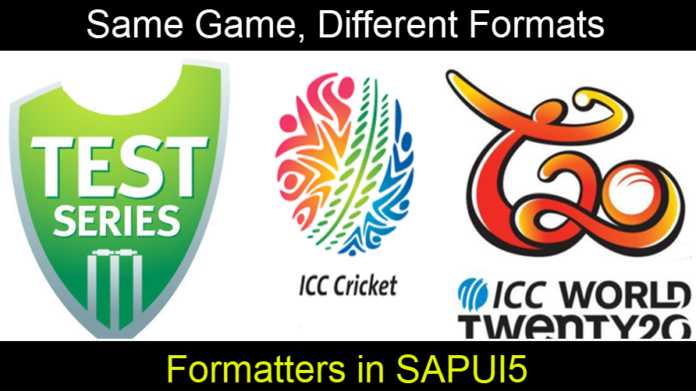
Formatter functions are used for formatting the data being rendered on various UI controls on the UI screen. For example, the data being fetched from backend via a Odata Model returns flag indicators like 0,1,2 etc. And you want to display a meaningful text to the end user corresponding to the flag. In this case, formatter function will be invoked and it will format the flag to a meaningful text shown to the end user.
In this tutorial, we will work through an example of formatter function. We will create a JSON Model and Bind one of its properties which contains codes/flag values to a Text Field on the UI and then we will define a formatter function and attach that function to the Text Field on the UI. Within the definition of formatter function, we will write logic to return corresponding meaningful text for a given input flag/code.
1. Create JSON Model
var oModel = new sap.ui.model.json.JSONModel();
oModel.setData({code:"1"});
sap.ui.getCore().setModel(oModel);
2. Create Text View in the View file
var oTextView = new sap.ui.commons.TextView("textView", {
// bind text property of textview to code property of model
text: "{/code}",
<strong>formatter: '.formatter.statusText'</strong>
});
3. Create Formatter.js file
Create formatter.js file in your WebContent/model folder. Write below code in it. We are letting the application know what to return when 0, 1 or 2 is received.
sap.ui.define([], function () {
"use strict";
return {
statusText: function (sStatus) {
switch (sStatus) {
case "0":
return 'New';
case "1":
return 'In Progress';
case "2":
return 'Completed';
default:
return sStatus;
}
}
};
});
4. Refer formatter.js file in View’s Controller
Define
sap.ui.define([
"sap/ui/core/mvc/Controller",
"sap/ui/model/json/JSONModel"<strong>,
"sap/ui/demo/wt/model/formatter"</strong>
], function (Controller, JSONModel, <strong>formatter</strong>) {
"use strict";
return Controller.extend("org.test.controller.Sample", {
<strong>formatter: formatter,</strong>
Output
With this coding done, below output will be displayed on the screen.
When ‘code’ property value is 0, output will be ‘New’ as per formatter function definition in step 3 above.
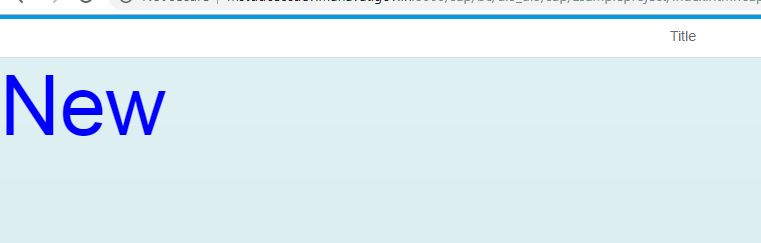
When ‘code’ property value is 1, output will be ‘In Progress’ as per formatter function definition
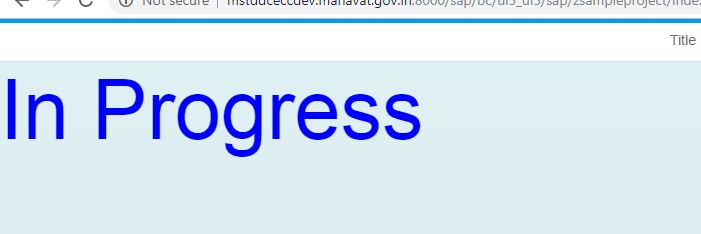
When ‘code’ property value is 2, output will be ‘Completed’ as per formatter function definition
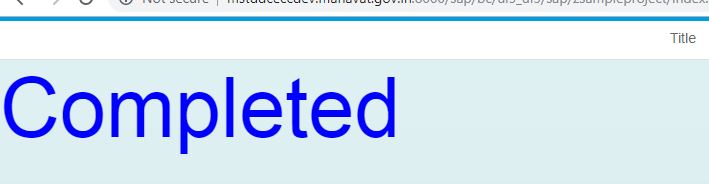
This is a simple demonstration of formatter function in SAPUI5. If you have a very complex formatting to be done, the steps remain the same. Formatters are very useful in numerous real project scenarios like controlling the color of output or visibility of an element and many more.