I’ll explain how to add (Me21n/Me22n/Me23n) customer fields for sales order on item basis.
1. Adding a New Field to the Database
i) Go to the CI_EKPODB table from the Se11 transaction code and add new field with the Append Structure button.
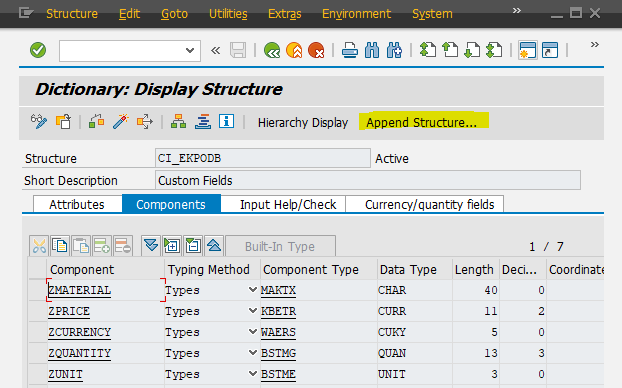
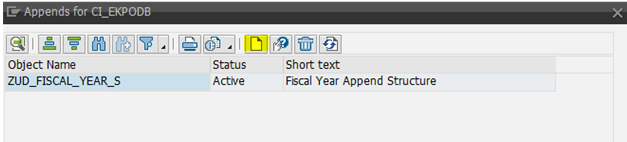
ii) After activating the created Append Structure, the field will be added to the CI_EKPODB table.
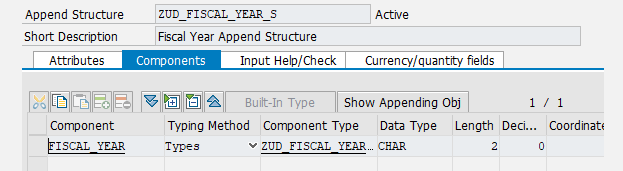
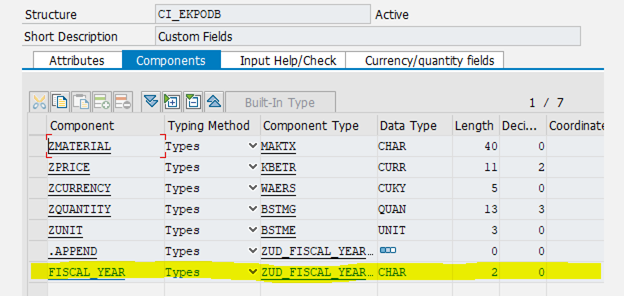
2. Creating Tables and Structures to Use
i) From transaction code Se11 create a table like this;
- MANDT
- EBELN
- EBELP
- NEW FIELD
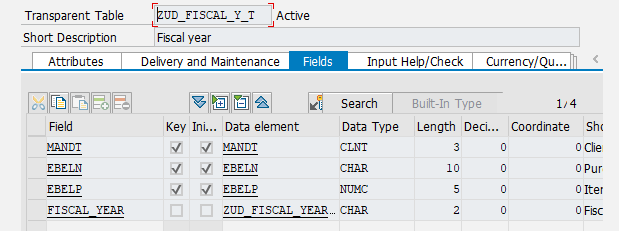
ii) From transaction code Se11 create a structure like this;
NEW FIELD
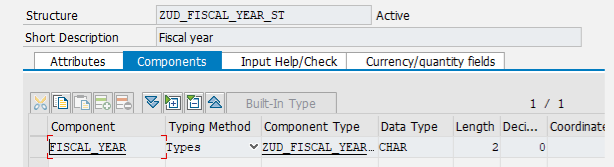
3. Creating the Screen to Add to the Tab
i) A copy of the SAPLMEPOBADIEX Function Pool program from the SAP sample programs is created from the Se38 transaction code.
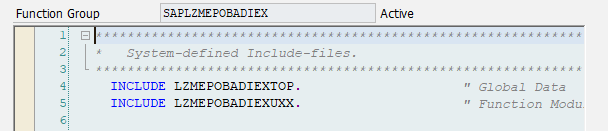
ii) Create a screen for new field in the Function Pool program that created in the Se51 transaction code.
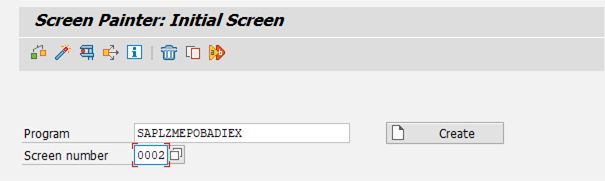
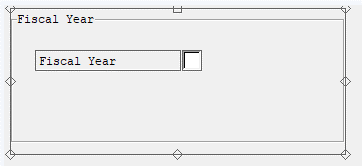
-> Attention should be paid to the created screen is subscreen in the attributes tab.
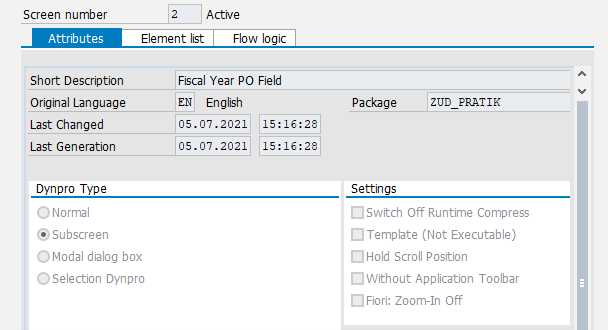
-> Event_pbo and event_pai modules are created from the Flow Logic tab.
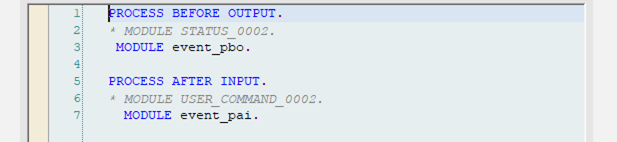
iii) Organize the global data in the top include of the Function Pool program as follows.

-> In lines 4 and 8, new definitions are given to the table created in Step 2.
-> In line 2, the structure created in Step 2 is given.
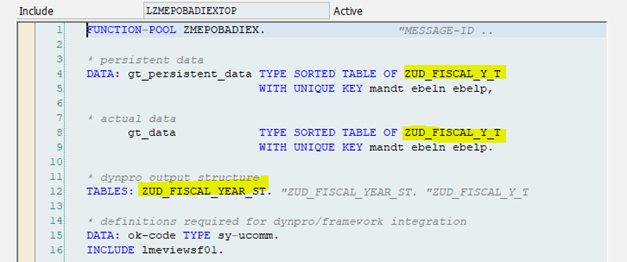
iv) Function Modules are organized by taking samples from the SAPLMEPOBADIEX Function Pool program that we copied.
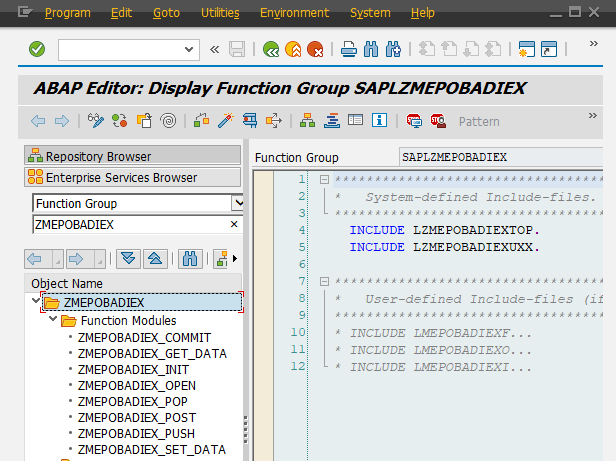
a) MEPOBADIEX_GET_DATA
Import Parameter
- IM_EBELN type EBELN
- IM_EBELP type EBELP
Export parameter
1. EX_DATA type the table created in Step 2
-> The module copied for Source Code is used exactly, and the data of the field want to add is added by pulling it.
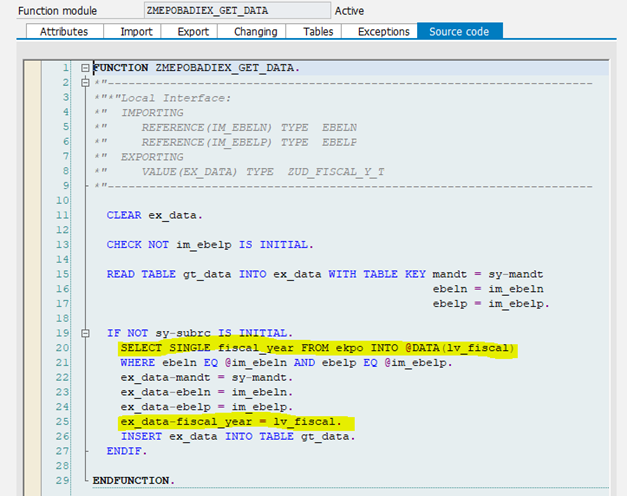
CLEAR ex_data.
CHECK NOT im_ebelp IS INITIAL.
READ TABLE gt_data INTO ex_data WITH TABLE KEY mandt = sy-mandt
ebeln = im_ebeln
ebelp = im_ebelp.
IF NOT sy-subrc IS INITIAL.
SELECT SINGLE fiscal_year FROM ekpo INTO @DATA(lv_fiscal)
WHERE ebeln EQ @im_ebeln AND ebelp EQ @im_ebelp.
ex_data-mandt = sy-mandt.
ex_data-ebeln = im_ebeln.
ex_data-ebelp = im_ebelp.
ex_data-fiscal_year = lv_fiscal.
INSERT ex_data INTO TABLE gt_data.
ENDIF.
b) MEPOBADIEX_PUSH
Import Parameter
1. IM_DYNP_DATA type the structure created in Step 2
-> Source Code: Filled with the structure created in Step 2.
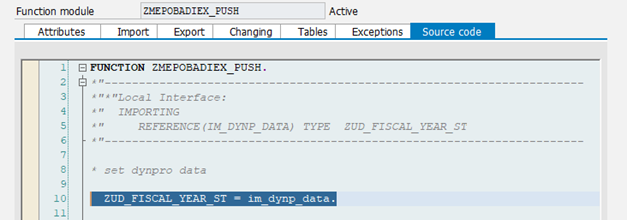
ZUD_FISCAL_YEAR_ST = im_dynp_data.
c) MEPOBADIEX_POP
Export Parameter
1. EX_DYNP_DATA type 2. Adımdaki Structure
-> Source Code: Export parameter filled with the structure created in Step 2.
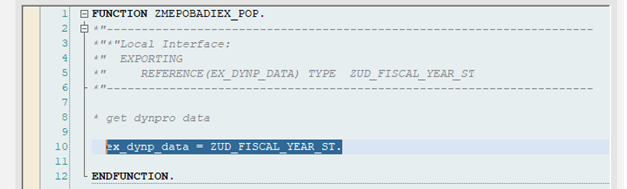
ex_dynp_data = ZUD_FISCAL_YEAR_ST.
d) MEPOBADIEX_SET_DATA
-> Source Code; On line 30 it is mapped to new field.
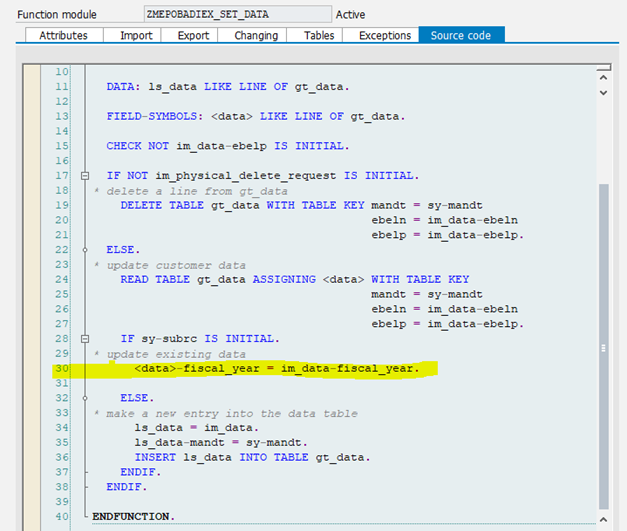
DATA: ls_data LIKE LINE OF gt_data.
FIELD-SYMBOLS: <data> LIKE LINE OF gt_data.
CHECK NOT im_data-ebelp IS INITIAL.
IF NOT im_physical_delete_request IS INITIAL.
DELETE TABLE gt_data WITH TABLE KEY mandt = sy-mandt
ebeln = im_data-ebeln
ebelp = im_data-ebelp.
ELSE.
READ TABLE gt_data ASSIGNING <data> WITH TABLE KEY
mandt = sy-mandt
ebeln = im_data-ebeln
ebelp = im_data-ebelp.
IF sy-subrc IS INITIAL.
<data>-fiscal_year = im_data-fiscal_year.
ELSE.
ls_data = im_data.
ls_data-mandt = sy-mandt.
INSERT ls_data INTO TABLE gt_data.
ENDIF.
ENDIF.
4. BadI Implemantation
i) From Se18 transaction code to ME_GUI_PO_CUST (classical) BadI;
Display -> Implementation -> Overview
-> If it does not exist, it is created or the existing one is used.
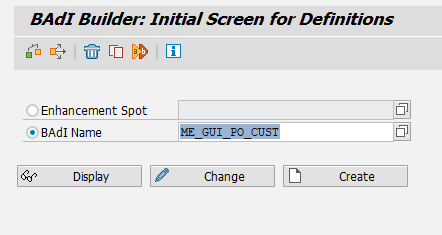
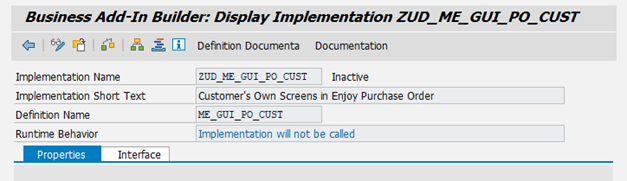
ii) Changes are made for the class.

Attribute:
- SUBSCREEN1 / Level: Constant / Visibility: Public / Type: MEPO_NAME / Initial Value ‘ITEMSCREEN1’
- DYNP_DATA_PBO / Level Instance Attribute / Visibility Private / Associated Type the structure created in Step 2
- DYNP_DATA_PAI / Level Instance Attribute / Visibility Private / Associated Type the structure created in Step 2

iii) Necessary changes are made to show our new tab in the SUBSCRIBE method.
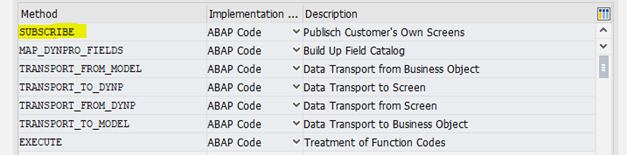
ls_subscriber-name = Attribute created
ls_subscriber-dynpro = Number of Screen Created in Se51
ls_subscriber-program = Name of Function Pool Program
ls_subscriber-struct_name = Structure in Step 2
ls_subscriber-label = Name to Display in Tab
ls_subscriber-position = Tab Position
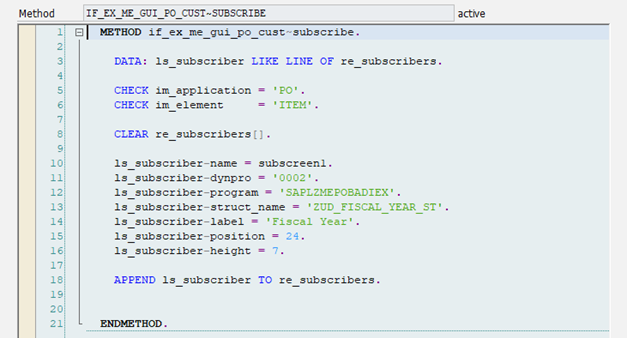
DATA: ls_subscriber LIKE LINE OF re_subscribers.
CHECK im_application = 'PO'.
CHECK im_element = 'ITEM'.
CLEAR re_subscribers[].
ls_subscriber-name = subscreen1.
ls_subscriber-dynpro = '0002'.
ls_subscriber-program = 'SAPLZMEPOBADIEX'.
ls_subscriber-struct_name = 'ZUD_FISCAL_YEAR_ST'.
ls_subscriber-label = 'Fiscal Year'.
ls_subscriber-position = 24.
ls_subscriber-height = 7.
APPEND ls_subscriber TO re_subscribers.
iv) MAP_DYNPRO_FIELDS Necessary changes are made in the method.
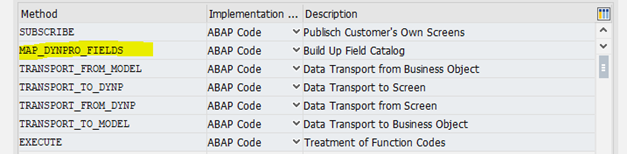
-> In line 7, add the new field
-> In line 8, add the mapping field
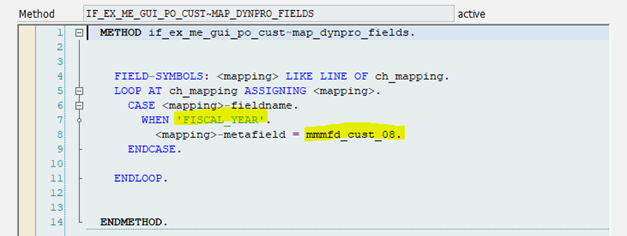
FIELD-SYMBOLS: <mapping> LIKE LINE OF ch_mapping.
LOOP AT ch_mapping ASSIGNING <mapping>.
CASE <mapping>-fieldname.
WHEN 'FISCAL_YEAR'.
<mapping>-metafield = mmmfd_cust_08.
ENDCASE.
ENDLOOP.
v) TRANSPORT_FROM_MODEL Necessary changes are made in the method.
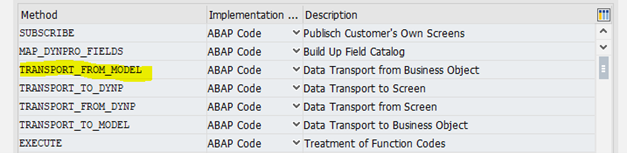
-> In line 23, Add the created Function Module.
-> In line 32, Do the necessary changes for the new field
DATA: l_item TYPE REF TO if_purchase_order_item_mm,
ls_mepoitem TYPE mepoitem,
ls_customer TYPE ZUD_FISCAL_Y_T.
CASE im_name.
WHEN subscreen1.
mmpur_dynamic_cast l_item im_model.
CHECK NOT l_item IS INITIAL.
ls_mepoitem = l_item->get_data( ).
CALL FUNCTION 'ZMEPOBADIEX_GET_DATA'
EXPORTING
im_ebeln = ls_mepoitem-ebeln
im_ebelp = ls_mepoitem-ebelp
IMPORTING
ex_data = ls_customer.
MOVE-CORRESPONDING ls_mepoitem TO dynp_data_pbo.
MOVE ls_customer-fiscal_year TO dynp_data_pbo-fiscal_year.
WHEN OTHERS.
ENDCASE.
vi) TRANSPORT_TO_DYNP Necessary changes are made in the method.
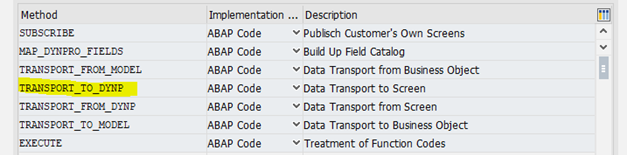
-> In line 7, Add the created Function Module.
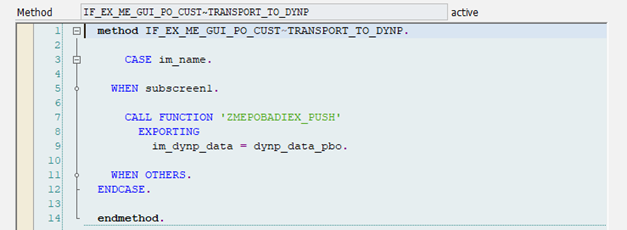
CASE im_name.
WHEN subscreen1.
CALL FUNCTION 'ZMEPOBADIEX_PUSH'
EXPORTING
im_dynp_data = dynp_data_pbo.
WHEN OTHERS.
ENDCASE.
vii) TRANSPORT_FROM_DYNP Necessary changes are made in the method.
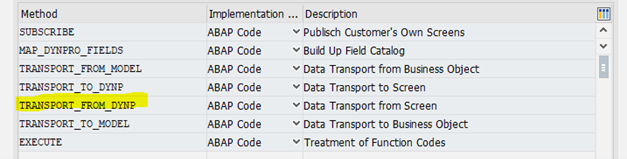
-> In line 7, Add the created Function Module.
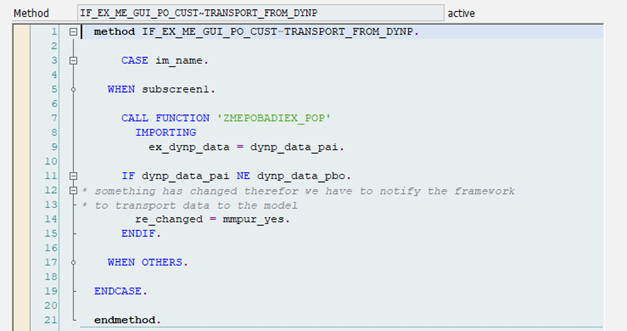
CASE im_name.
WHEN subscreen1.
CALL FUNCTION 'ZMEPOBADIEX_POP'
IMPORTING
ex_dynp_data = dynp_data_pai.
IF dynp_data_pai NE dynp_data_pbo.
re_changed = mmpur_yes.
ENDIF.
WHEN OTHERS.
ENDCASE.
viii) TRANSPORT_TO_MODEL Necessary changes are made in the method.
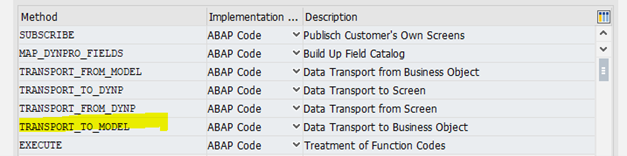
-> In line 21, 31 and 37, Do the necessary changes for the new field
-> In line 24 and 33, Add the created Function Module.
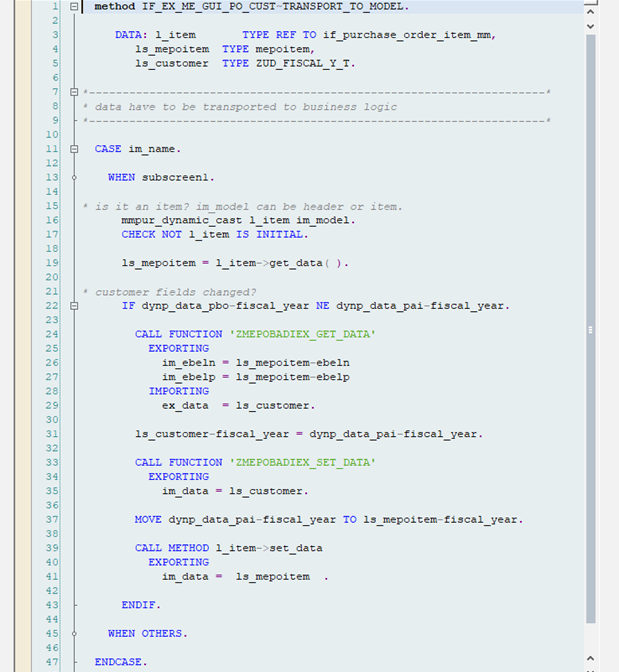
DATA: l_item TYPE REF TO if_purchase_order_item_mm,
ls_mepoitem TYPE mepoitem,
ls_customer TYPE ZUD_FISCAL_Y_T.
CASE im_name.
WHEN subscreen1.
mmpur_dynamic_cast l_item im_model.
CHECK NOT l_item IS INITIAL.
ls_mepoitem = l_item->get_data( ).
IF dynp_data_pbo-fiscal_year NE dynp_data_pai-fiscal_year.
CALL FUNCTION 'ZMEPOBADIEX_GET_DATA'
EXPORTING
im_ebeln = ls_mepoitem-ebeln
im_ebelp = ls_mepoitem-ebelp
IMPORTING
ex_data = ls_customer.
ls_customer-fiscal_year = dynp_data_pai-fiscal_year.
CALL FUNCTION 'ZMEPOBADIEX_SET_DATA'
EXPORTING
im_data = ls_customer.
MOVE dynp_data_pai-fiscal_year TO ls_mepoitem-fiscal_year.
CALL METHOD l_item->set_data
EXPORTING
im_data = ls_mepoitem .
ENDIF.
WHEN OTHERS.
ENDCASE.
4. BadI Enhancement
i) Enhancement is done to ME_PROCESS_PO_CUST (enhancement spot) BadI from Se18 transaction code.
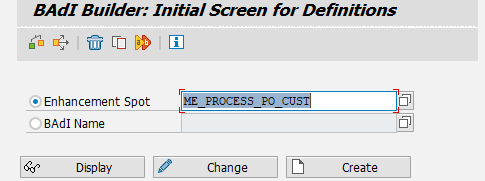
-> For example codes, the class named CL_EXM_IM_ME_PROCESS_PO_CUST is used.
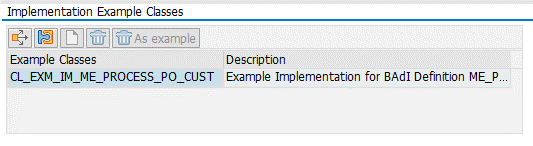
-> A new enhancement is made or an existing one is used.
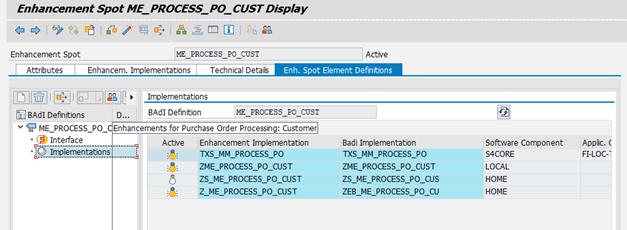
ii) FIELDSELECTION_ITEM Necessary changes are made in the method.
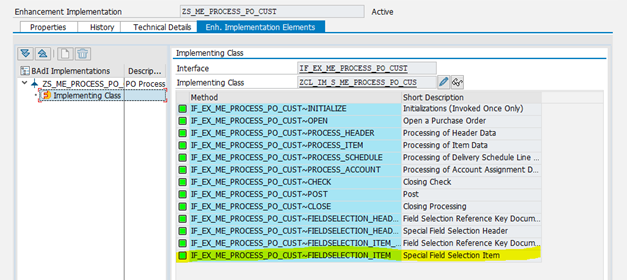
-> In line 22, add the mapping field
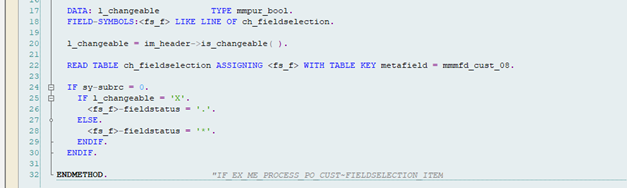
DATA: l_changeable TYPE mmpur_bool.
FIELD-SYMBOLS:<fs_f> LIKE LINE OF ch_fieldselection.
l_changeable = im_header->is_changeable( ).
READ TABLE ch_fieldselection ASSIGNING <fs_f> WITH TABLE KEY metafield = mmmfd_cust_08.
IF sy-subrc = 0.
IF l_changeable = 'X'.
<fs_f>-fieldstatus = '.'.
ELSE.
<fs_f>-fieldstatus = '*'.
ENDIF.
ENDIF.
iii) PROCESS_ITEM Necessary changes are made in the method.
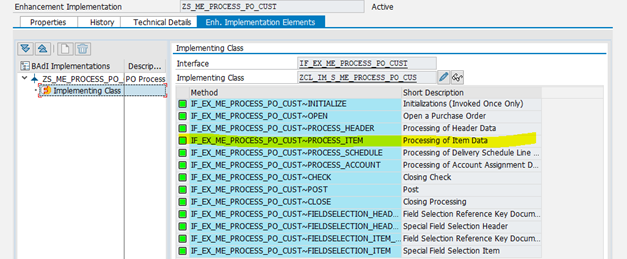
-> Make a selection according to the field added and edit the 94th line.
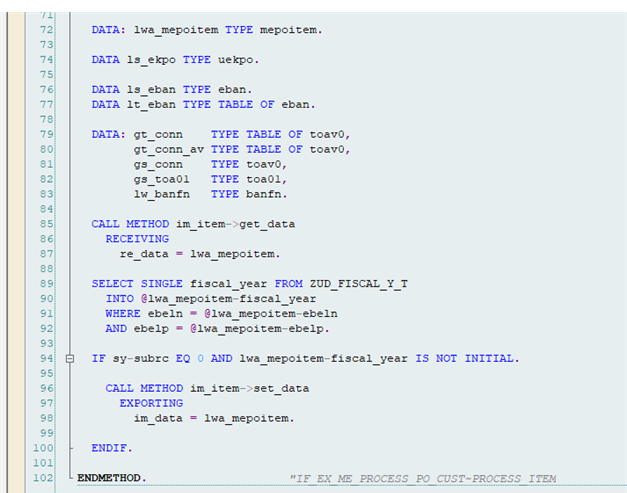
DATA: lwa_mepoitem TYPE mepoitem.
DATA ls_ekpo TYPE uekpo.
DATA ls_eban TYPE eban.
DATA lt_eban TYPE TABLE OF eban.
DATA: gt_conn TYPE TABLE OF toav0,
gt_conn_av TYPE TABLE OF toav0,
gs_conn TYPE toav0,
gs_toa01 TYPE toa01,
lw_banfn TYPE banfn.
CALL METHOD im_item->get_data
RECEIVING
re_data = lwa_mepoitem.
SELECT SINGLE fiscal_year FROM ZUD_FISCAL_Y_T
INTO @lwa_mepoitem-fiscal_year
WHERE ebeln = @lwa_mepoitem-ebeln
AND ebelp = @lwa_mepoitem-ebelp.
IF sy-subrc EQ 0 AND lwa_mepoitem-fiscal_year IS NOT INITIAL.
CALL METHOD im_item->set_data
EXPORTING
im_data = lwa_mepoitem.
ENDIF.