In this post, I am going to write about how can we clear the metadata cache for OData services. This feature can be useful for developers when we generate OData services using CDS and BOPF. When we add / delete / modify any property in the entity set or an annotation in the CDS or any BOPF functionality such as Creatable, Updatable or Deletable, then we need to clear the metadata cache for our changes to be visible in the Metadata.xml file of the OData service, which can then be consumed in any UI5 application.
To clear this OData Metadata Cache, the below two ways can primarily be used:
- Through the use of TCodes.
- Through coding in ABAP.
Let us look into the above methods.
Clearing of OData Metadata Cache by using TCodes:
In this method, we make use of the TCodes /n/iwfnd/maint_service and /n/iwfnd/gw_client to clear our metadata cache. Follow the below steps:
Go to the TCode /n/iwfnd/maint_service and from the list of services select your desired service. You can also filter the service with its technical name using the filter button.
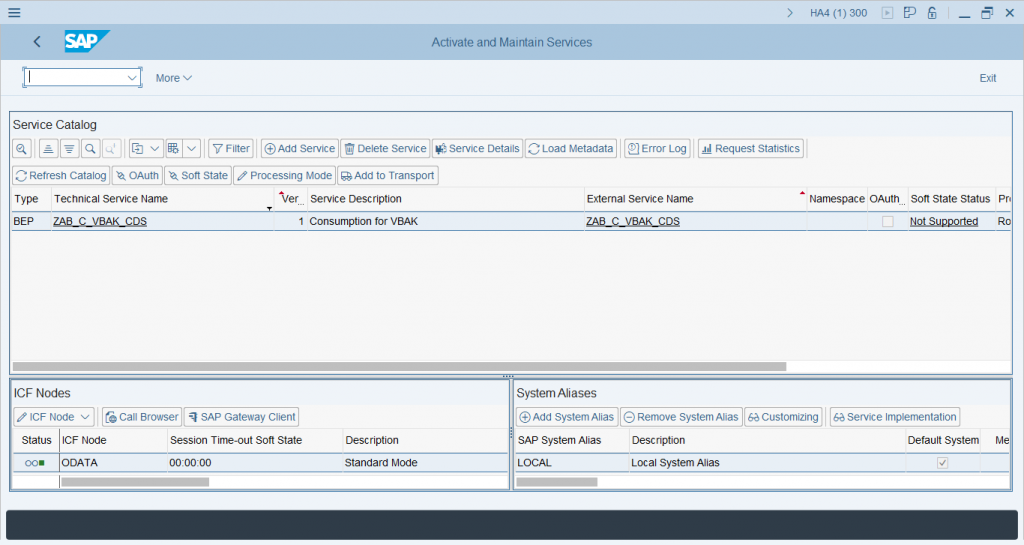
Now, for the above service, to clear its metadata, we can go to the TCode /n/iwfnd/gw_client or click on ths SAP Gateway Client button on the ICF Nodes Section in the bottom left hand corner.
The below screen comes up:
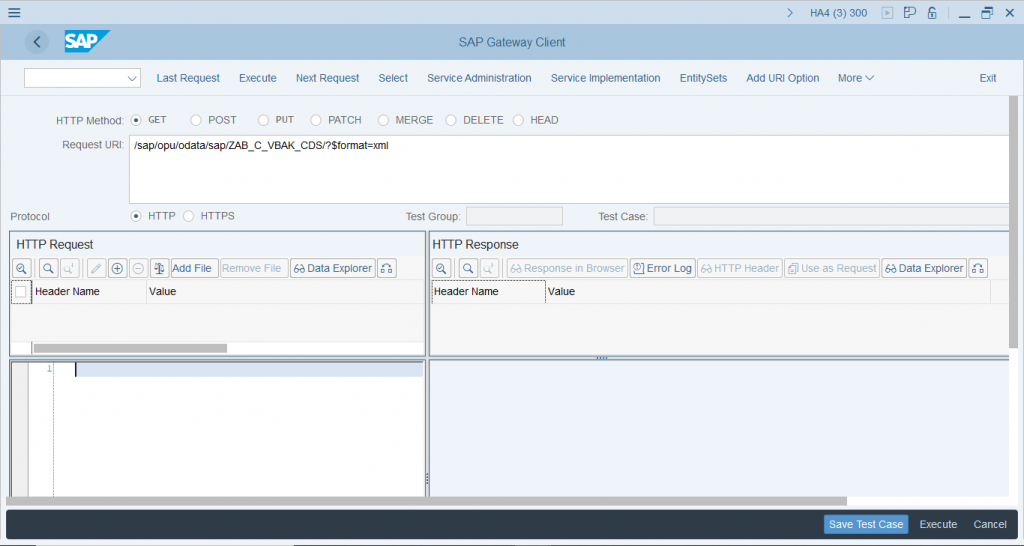
Now, to clear the metadata cache, we can click on more, in the popup menu, select metadata, then select on Clean up Cache and in the next popup, click on the “On Both Systems” option.
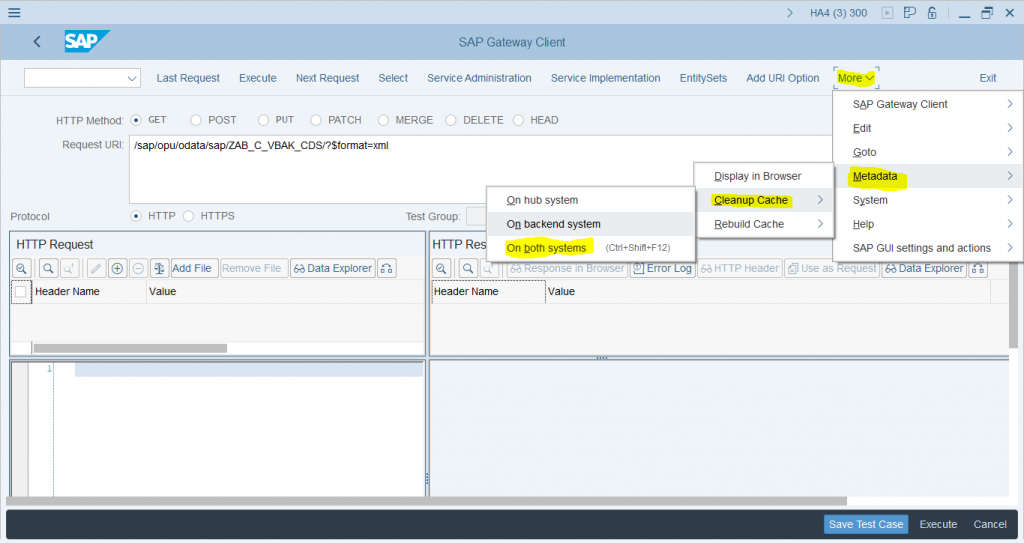
This can be done using a shortcut key combination : Ctrl + Shift + F12.
On performing the above step, if the cache is successfully cleared, the below success message is displayed:

Variations due to type of systems:
As we know, there are basically two types of implementation of SAP FIORI & NetWeaver Gateway, one is Embedded Implementation, where both the front end system (NetWeaver Gateway i.e. OData and FIORI) and the back end System (ECC/Hana) are on the same server. The other one is Hub implementation, where the NetWeaver Gateway and the ECC/Hana resides in two different servers and they communicate with each other through RFC calls.
To clear the metadata cache using the above process, for the Embedded implementation, it is enough to clear only on the back end system, but in Hub implementation, it is mandatory to clear the cache on both systems to properly clear the metadata cache.
Another way to clear the metadata cache is with the tcode /n/IWBEP/REG_SERVICE.
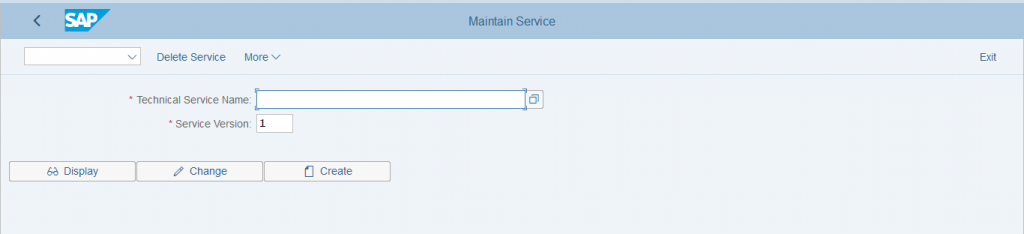
Here, we can give the Technical Service Name of our service and click on the Display button.
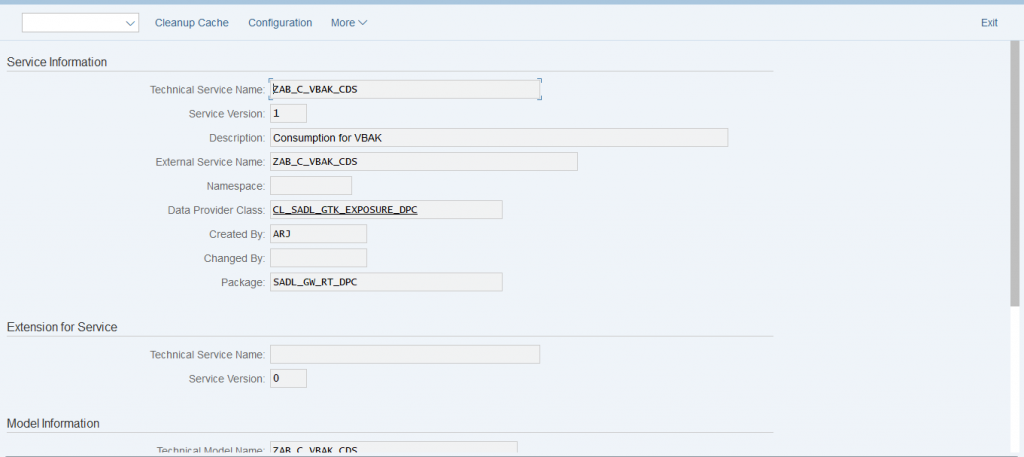
Here, we can click on the cleanup cache button and our service’s metadata cache will get deleted.
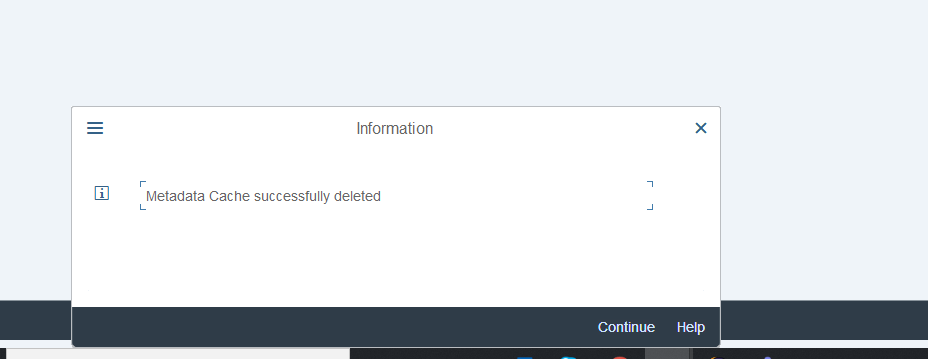
Apart from cleaning of metadata cache, the TCode /n/IWBEP/REG_SERVICE can be used to configure our OData services. We can also get details of the Model Provider and Data Provider Class, the technical service name and other service details. We can also use this TCode to delete our service. It would delete all the classes related to our service also.
Clearing OData Metadata Cache through ABAP Coding:
In this method, we can place an piece of ABAP code in any report or function module or class method and execute it whenever we wish to clear the cache of the Metadata of our OData Service. Below is an SE38 report with the code snippet that can be used to clear the metadata cache.
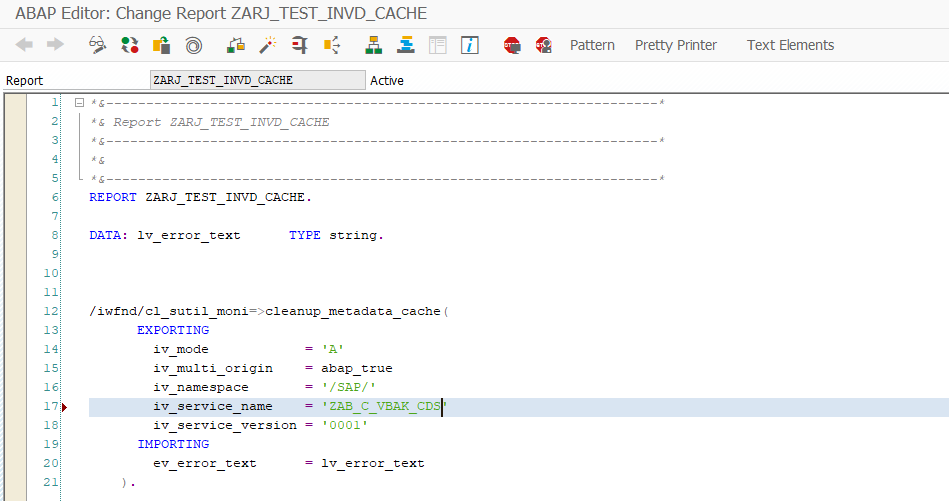
Here’s the code:
DATA: lv_error_text TYPE string.
/iwfnd/cl_sutil_moni=>cleanup_metadata_cache(
EXPORTING
iv_mode = 'A'
iv_multi_origin = abap_true
iv_namespace = '/SAP/'
iv_service_name = 'Your Service Name'
iv_service_version = '0001'
IMPORTING
ev_error_text = lv_error_text
).
Here, we are calling a method “cleanup_metadata_cache” from the /IWFND/CL_SUTIL_MONI class. This class is primarily used to work with the configurations of our OData Service.
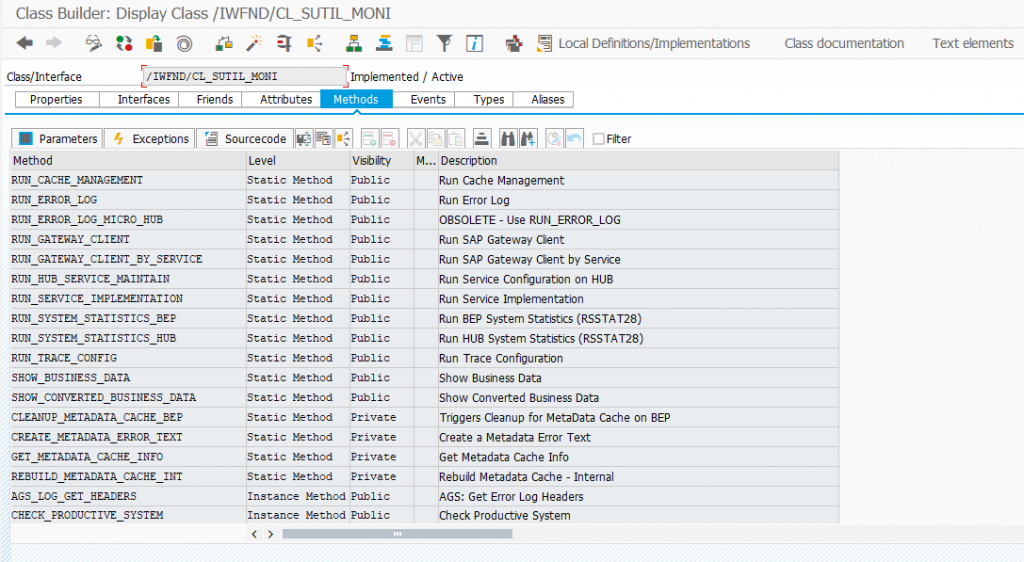
There are many other methods at our disposal, which we can use as per our requirement.
The cleanup metadata cache takes up some exporting parameters such as:
iv_mode – We have options such as
- A – Clean cache on both systems
- B – Clean cache only on backend system
- C – Clean cache only on hub system
iv_multi_origin – We set this as true as our service could use multiple systems.
iv_namespace – This is the namespace of our service. As we know the mostly all our OData service is stored under the ‘/SAP/’ namespace (Can be checked from SICF TCode), we can set the same to this parameter.
iv_service_name – Here we can place our service name (Use the technical service name).
iv_service_version – Here we can place our service version. Usually it is ‘0001’. Only in some rare cases it is otherwise.
One thing to add here, is in the previous approach, where we have used the /n/iwfnd/gw_client TCode to clear the cache, it internally uses this class only. If we set a break point for click of the button “On both systems” in the menu, we can check the entire flow of how the metadata cache gets cleared.
To get more information on this, go to the TCode se80 and check out the program “/IWFND/SUTIL_GW_CLIENT”, and the screen 0110 in particular. It contains most of the code for clearing the metadata cache, such as fetching the service name and version during the run time dynamically with the use of function modules.
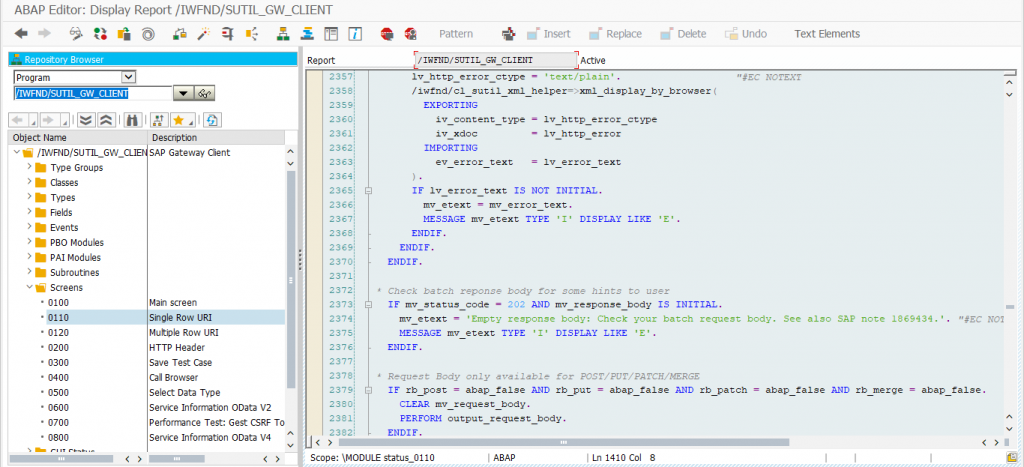
Using this method, we can add the above code snippet to clear the cache anywhere we wish in the system, such as inside the get_entityset method, or any function modules, class methods, reports, standalone programs, or even inside an BOPF determination. In any scenario if we require the metadata of the OData Service to be cleared after any operation from the UI, we can create a determination to the BOPF, and place our code there.
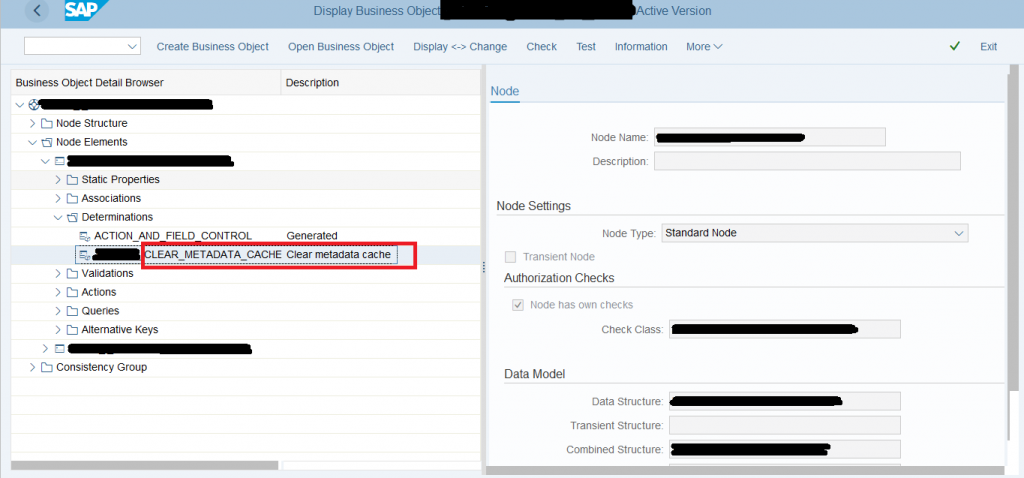
The metadata cache will be cleared after every UI operation. BOPF Determination code can be written like below:
method /BOBF/IF_FRW_DETERMINATION~EXECUTE.
data: lt_serv_data type "Enter the type from the Types tab in the class.
io_read->RETRIEVE(
EXPORTING
IV_NODE = "Enter the bofp node name here
IT_KEY = it_key " Key Table
IV_FILL_DATA = ABAP_TRUE " Data element for domain BOOLE: TRUE (='X') and FALSE (=' ')
IMPORTING
ET_DATA = lt_serv_data " Data Return Structure
).
if lt_serv_data is NOT INITIAL.
DATA: lv_error_text TYPE string,
lv_serv type /iwfnd/ui_service_name ."value lt_serv_data [ 1 ]-SERVICE_NAME.
lv_serv = lt_serv_data[ 1 ]-SERVICE_NAME.
/iwfnd/cl_sutil_moni=>cleanup_metadata_cache(
EXPORTING
iv_mode = 'A'
iv_multi_origin = abap_true
iv_namespace = '/SAP/'
iv_service_name = lv_serv
iv_service_version = '0001'
IMPORTING
ev_error_text = lv_error_text
).
ENDIF.
endmethod.
So, from the above code, we can dynamically get the operation, keys and other UI stuff by retrieving it from the BOPF call and then clear the cache.
Note: How do we make sure that, our OData service’s metadata cache has been actually cleared?
The metadata of our OData service is generated from the Model Provider Class. Primarily the DEFINE method in the MPC class. So, we can go to this DEFINE method of our OData service project in SEGW TCode and place a break point inside the DEFINE method.
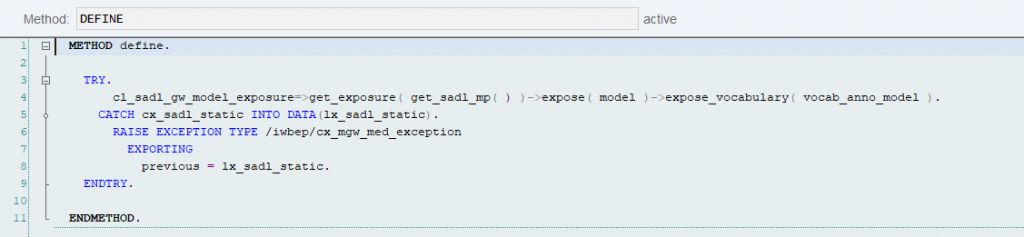
When the metadata of the service is requested for the first time, the MPC class will generate it and it will be placed in the system cache. So from the second time onwards when the metadata is requested, it will be brought from the cache and not generated from the MPC class.
But, when we invalidate the metadata cache of the service, and then request for the metadata, it will have to be generated by the MPC class again, therefore, the break point in the DEFINE method will get triggered.
So, if we place a break point in the DEFINE method of out MPC class, based on its triggering, we can come to know if our service’s metadata is actually cleared.
Note: How can we navigate to the Model Provider Class of a OData Service that has been directly generated from the CDS view instead of using the SADL method ?
In case the OData service is directly generated from the CDS view, we can go to the TCode /n/IWBEP/REG_SERVICE, give our CDS generated OData Service name and click on display.
In the next screen, we can scroll down to the Model Information section and double click on the Model Provider Class mentioned in the form.
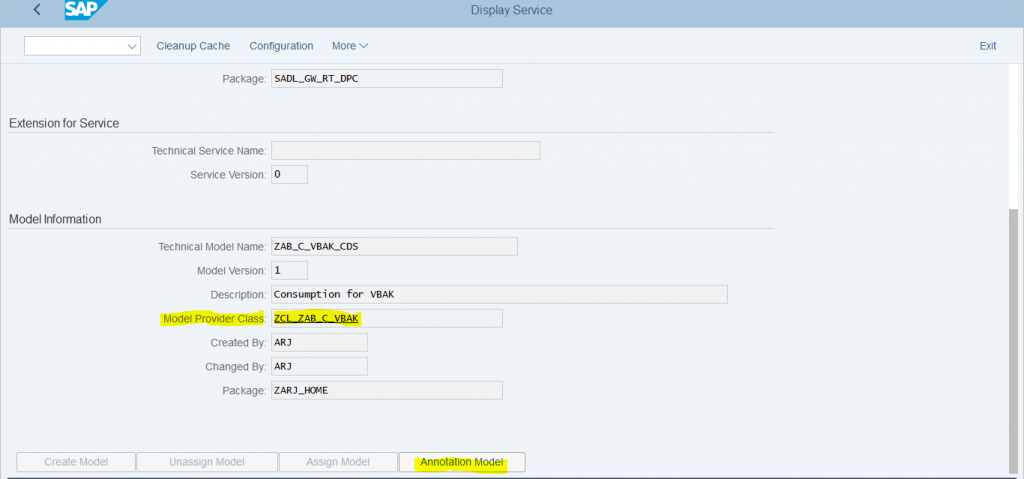
This TCode can also be used to check the annotations for our service, which can later be used in our UI5 application. We can do this by clicking on the Annotation Model button in the above screen.
Note: In what ways can we ensure to clear any OData based / Metadata Cache from our UI5 application ?
To clear any back end cache and receive updated information from back end, we can follow some basic steps.
Firstly, open the manifest.json in the WebIDE, in Descriptor Editor Format. Navigate to Data Sources and click on the Sync Metadata Button.
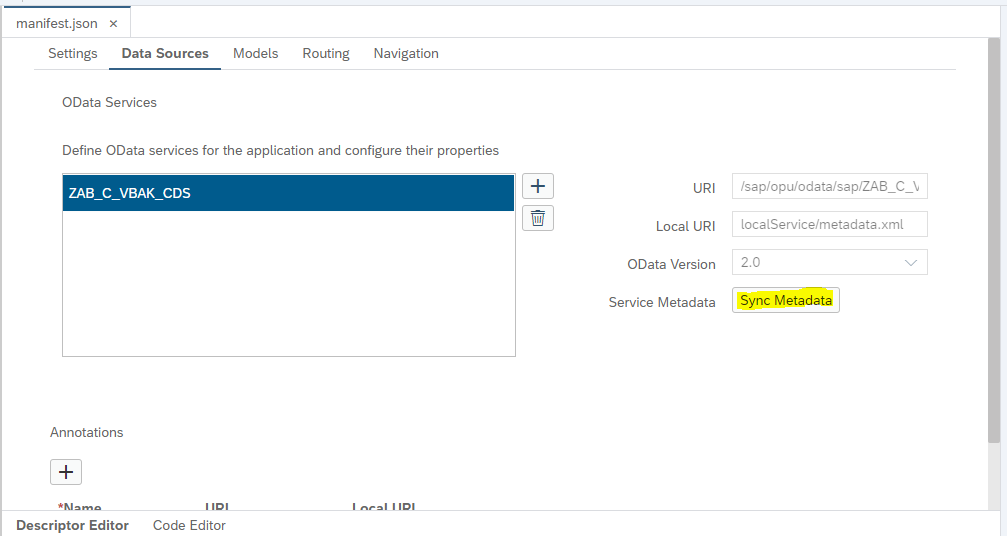
It would sync your locally stored metadata in the UI5 app (in case you are using one) to the actual OData service metadata.
Secondly, we can also do empty cache and hard reload by running the application.
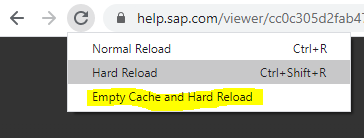
Note:
The above processes is used for clearing the Metadata Cache only. These processes might not be effective in clearing of OData Service Cache and BSP application Cache. There are other dedicated methods available to clear these caches .