Introduction
There are several blogs showing how to organize interaction between SAC and S/4HANA using OData services. However, one cannot ignore the fact that SAC, as an analytical tool, often uses data from SAP BW or BW/4HANA. This blog provides a simple example of how an OData Service can be used to exchange information between a SAC Analytic Application and BW over a live connection. It is important to note, that this exchange is bi-directional.
Our scope does not include a detailed discussion of the rich features of OData, such as the use of complex types in services. Only the necessary setup steps are shown. If necessary, a specialist will be able to use much more complex Services, required in practical tasks, following to the same scheme.
It should also be noted that described solution can be used to transfer data from SAC to BW with subsequent processing in ABAP. This may be required in cases where the SAC scripting language capabilities are insufficient for complex calculations. Also, this solution is useful if the SAC Analytic Application is used as a replacement for the Web Dynpro applications, where it was possible to initiate the execution of any actions at the Application Server using standard ABAP tools.
Prerequisites
CORS Settings
The described scheme only works when using a live connection between SAC and BW. When creating this connection, BASIS Team performs CORS settings on the AS ABAP side.
Authorizations
It is assumed that the developer of the OData service has the standard set of ABAP development permissions. Including creating and editing ABAP classes and using the debugger. Additionally, following permissions will be required:
– transaction SEGW
– transactions /IWFND/***
– transactions /IWBEP/***
These authorizations can be described as follows:

– SEGW development authorization: authorization object /IWBEP/SB. In our example, a project (i.e. OData service) with the technical name ZODATAV4 will be created, it will be placed in the local ABAP package $TMP. Corresponding authorization should look as follows:

– authorizations to start the OData service. In our example, ZODATAV4_GROUP service group will be created. To do this, you will first need to have the following authorizations on the S_USER_STA object:
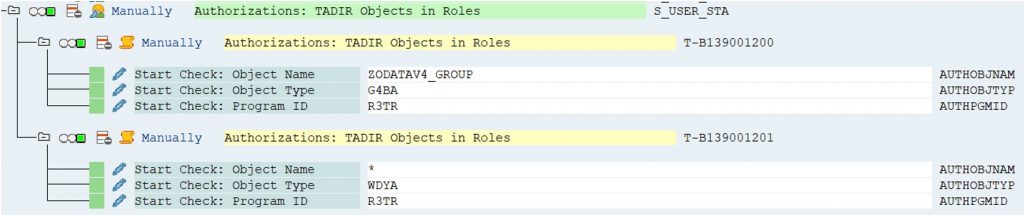
Here the choice is made from the list in the AUTHOBJNAM field. Please, note that you must first select the type of object in the “Type” drop-down list
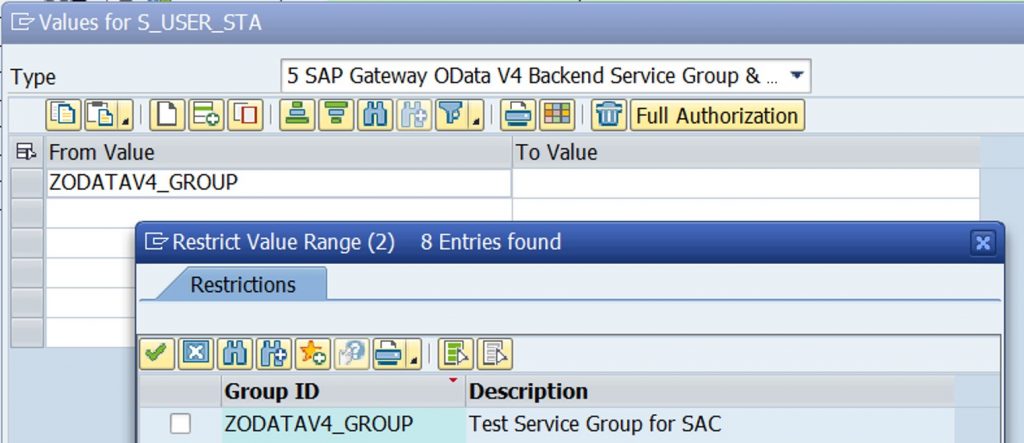
Then the required values can be selected from the list or the “Full Authorization” button is pressed. After confirming the selection, AUTHOBJTYP and AUTHPGMID fields will be filled in automatically according to the value selected in the “Type” drop-down list.
After that you should restart transaction PFCG and add the following S_START authorization with the same way as previous:

S_USER_STA authorisations are required only while editing the S_START object. When it is done, S_USER_STA can be deactivated or removed from the role profile.
Demo Scenario
- OData Service creation and configuration in BW. For the service, an action will be defined that “is able to” launch a BW process chain.
- Creation of SAC Analytic Application.
- An OData connection will be placed in Application to communicate with our Service.
- A button will be placed in Application. An onClick-script for this button will call the action in OData Service.
OData Service creation
Press the “Create Project” button in SEGW transaction, enter project technical name and description. Make sure that “OData 4.0 Service” is selected as project type. Also, select a development package if necessary. Here and below, in all examples, objects are created locally in the $TMP package:
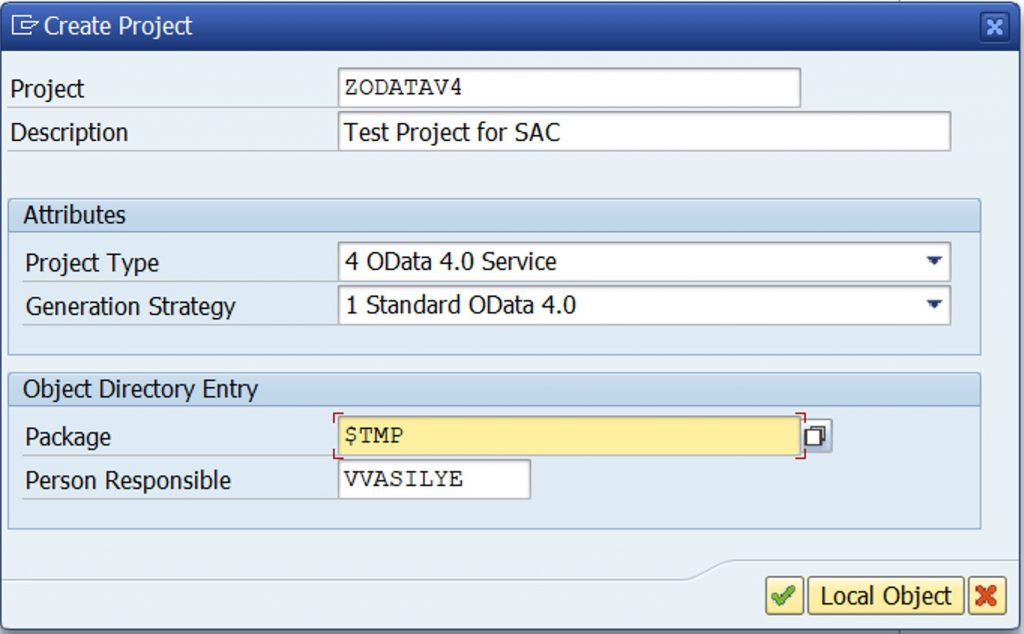
You can ignore following pop-up message:
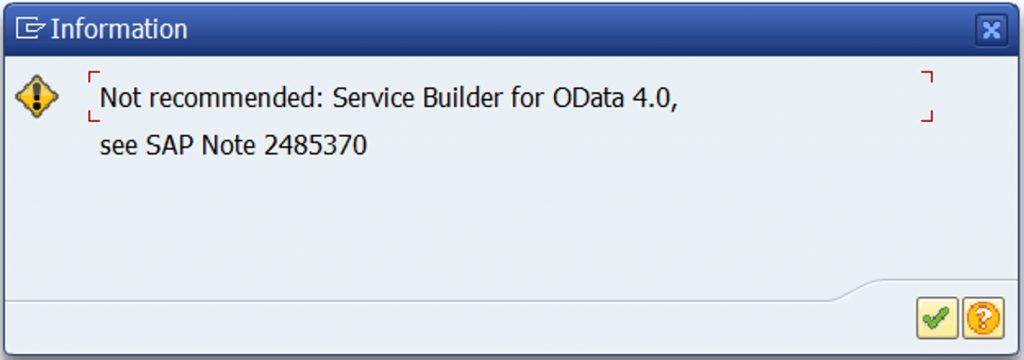
Select newly created Project and press the “Generate Runtime Objects” button:
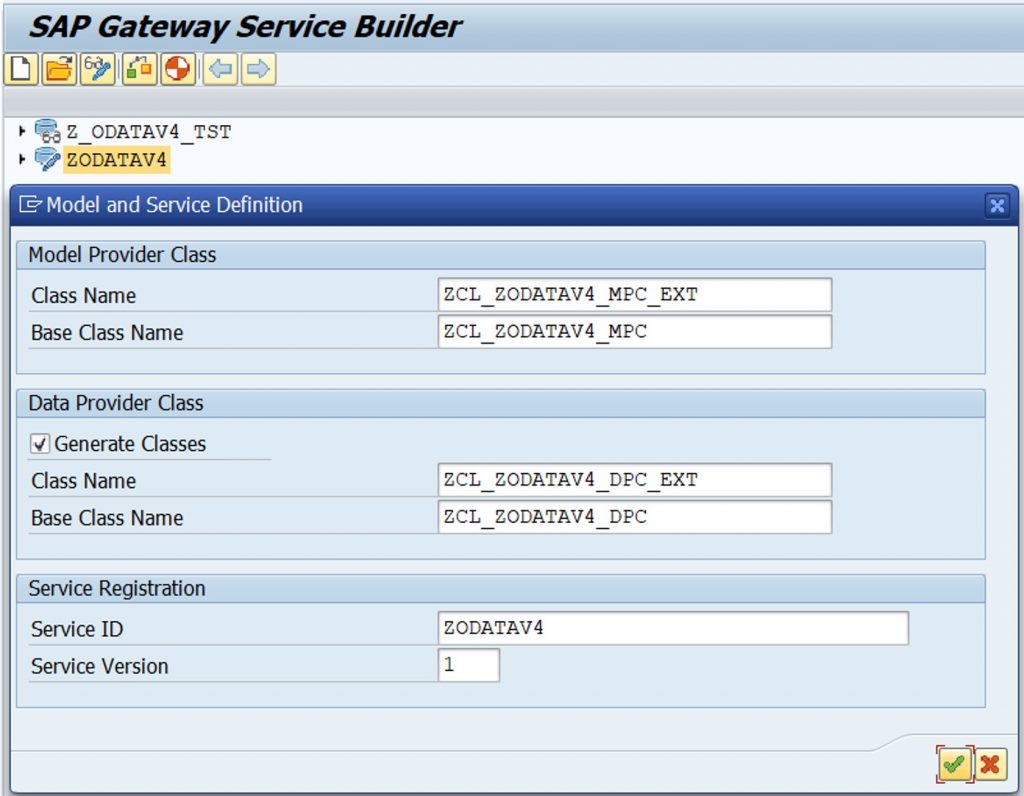
All fields in this window will be filled in automatically, you don’t have to change them.
The ZCL_ZODATAV4_MPC and ZCL_ZODATAV4_DPC classes contain standard code that is automatically copied by the system. This code should not be changed. All custom logic need to be implemented by descendant classes whose names end in _EXT.
The Model Provider Class will declare the actions available in OData Service. It also should describe the returns produced by actions. If necessary, you can declare input parameters for these actions. For our example, we will create a StartProcessChain action with a ChainTechName input parameter. The SAP BW process chain technical name will be passed to input parameter when the action is called. The action will have a string type return, which will show the ID of the chain run that was started during the action execution. This is an artificial example, only to demonstrate that input parameter and return of action are provide a bi-directional data exchange between SAC and BW.
To implement the logic described, we’ll create the new method START_CHAIN in Model Provider Class. Also, standard method /IWBEP/IF_V4_MP_BASIC~DEFINE should be redefined. Our action will be called directly from the SAC Analytic Application without instantiating any helper types. Therefore, it must be unbound, that is, described as an Action Import.
Let’s create the START_CHAIN method as follows:
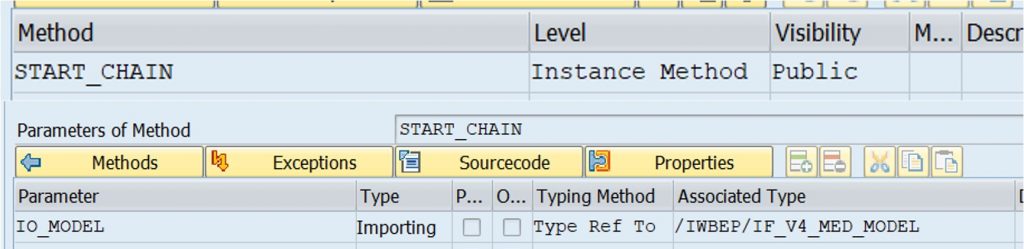
I added a code comments to clarify the logic:
METHOD start_chain.
DATA:
lo_action TYPE REF TO /iwbep/if_v4_med_action,
lo_action_import TYPE REF TO /iwbep/if_v4_med_action_imp,
lo_parameter TYPE REF TO /iwbep/if_v4_med_act_param,
lo_return TYPE REF TO /iwbep/if_v4_med_act_return.
"Declare an Action Import to be implemented in this OData Service
lo_action = io_model->create_action( 'STARTCHAIN' ).
lo_action->set_edm_name( 'StartProcessChain' ).
lo_action_import = lo_action->create_action_import( iv_action_import_name = 'STARTCHAIN' ).
lo_action_import->set_edm_name( 'StartProcessChain' ).
"Declare a local parameter type from standard OData types
io_model->create_primitive_type( iv_primitive_type_name = 'STRING' )->set_edm_type( /iwbep/if_v4_med_element=>gcs_edm_data_types-string ).
"Define an import parameter of this type for action described above
lo_parameter = lo_action->create_parameter( 'CHAIN' ).
lo_parameter->set_edm_name( 'ChainTechName' ).
lo_parameter->set_primitive_type( 'STRING' ).
"Declare that action should have a return and of which type it will be
lo_return = lo_action->create_return( ).
lo_return->set_primitive_type( 'STRING' ).
ENDMETHOD.
The redefined DEFINE method first calls the ancestor class method with the same input parameters. And then transfers control to the START_CHAIN method, specific to our task:
METHOD /iwbep/if_v4_mp_basic~define.
TRY.
CALL METHOD super->/iwbep/if_v4_mp_basic~define
EXPORTING
io_model = io_model
io_model_info = io_model_info.
CATCH /iwbep/cx_gateway .
ENDTRY.
start_chain( io_model ).
ENDMETHOD.
Data Provider Class implements the action return calculation logic. It will be enough to redefine the method /IWBEP/IF_V4_DP_ADVANCED~EXECUTE_ACTION for ZCL_ZODATAV4_DPC_EXT class. In our example, a standard function module will be used to start the chain in BW:
METHOD /iwbep/if_v4_dp_advanced~execute_action.
TYPES: BEGIN OF s_chain_action,
chain TYPE string,
END OF s_chain_action.
DATA: ls_chain_params TYPE s_chain_action,
l_chain TYPE rspc_chain,
l_logid TYPE rspc_logid,
l_response TYPE string.
DATA:
lv_action_name TYPE /iwbep/if_v4_med_element=>ty_e_med_internal_name,
ls_done TYPE /iwbep/if_v4_requ_adv_action=>ty_s_todo_process_list,
ls_todo TYPE /iwbep/if_v4_requ_adv_action=>ty_s_todo_list.
"Read todo list of requested operations
io_request->get_todos( IMPORTING es_todo_list = ls_todo ).
"Check that action import 'STARTCHAIN' was requested
IF ls_todo-process-action_import = abap_true.
io_request->get_action_import( IMPORTING ev_action_import_name = lv_action_name ).
IF lv_action_name = 'STARTCHAIN'.
io_request->get_parameter_data(
IMPORTING
es_parameter_data = ls_chain_params "Structure with the action parameters
).
ls_done-parameter_data = abap_true. "Parameters reading was executed
l_chain = ls_chain_params-chain. "This is necessary for data types compatibility
CALL FUNCTION 'RSPC_API_CHAIN_START'
EXPORTING
i_chain = l_chain
i_dont_wait = 'X'
i_gui = 'N'
IMPORTING
e_logid = l_logid.
l_response = l_logid.
io_response->set_busi_data( ia_busi_data = l_response ). "logid transferred as response of the action
ls_done-action_import = abap_true. "Action import was executed
io_response->set_is_done( ls_done ). "We return a list of executed operations
ENDIF.
ENDIF.
ENDMETHOD.
OData Service publishing
Service ZODATAV4 must be published in the system. Following steps are necessary for this:
- Create Service Group in transaction /IWBEP/V4_ADMIN, if there is no previously created group relevant for our purposes.
- Assign OData Service to Service Group.
- Publish Service Group, that is to make the services assigned to Group available for use by other users with the necessary authorizations (authorization object S_START). For that, the /IWFND/V4_ADMIN transaction is used.
Start transaction /IWBEP/V4_ADMIN and press the “Register Group” button. Enter Service Group technical name and description:
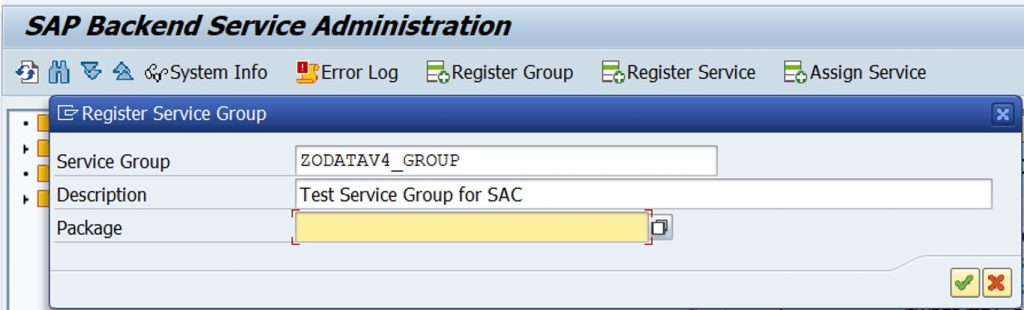
After creating a Group, the system will prompt you to immediately assign some Service to this Group:
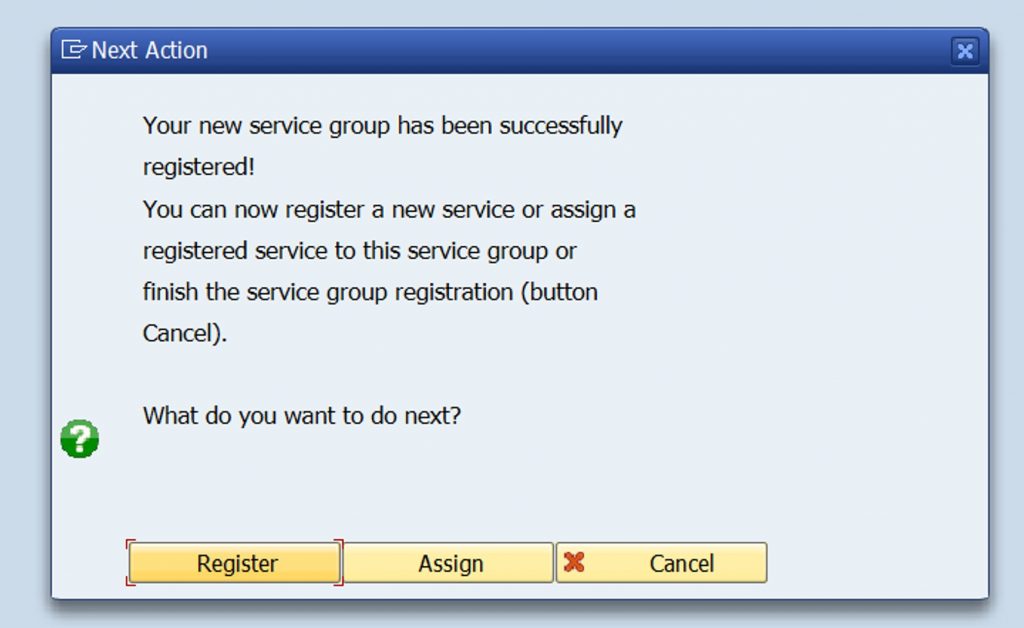
You can choose “Assign”. If the relevant Group has already been created earlier, or if you want to assign a Service to a Group later, you can do so using the “Assign Service” button. Here you can simply select a Service and a Group from the list:
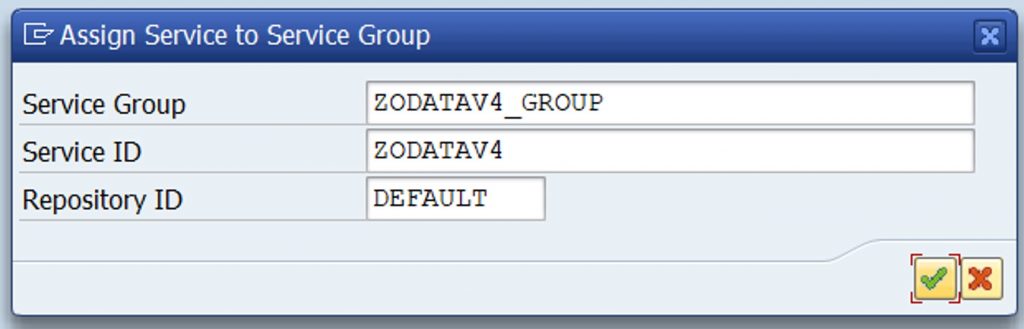
Service Group with the services assigned to it will be listed in the transaction:

If the Service Group has been newly created, then it must be published. To do this, go to transaction /IWFND/V4_ADMIN and click the “Publish Service Groups” button:

Since we are talking about objects in the current system, select LOCAL in the System Alias field, and then click “Get Service Groups”. A list of all unpublished groups will appear:
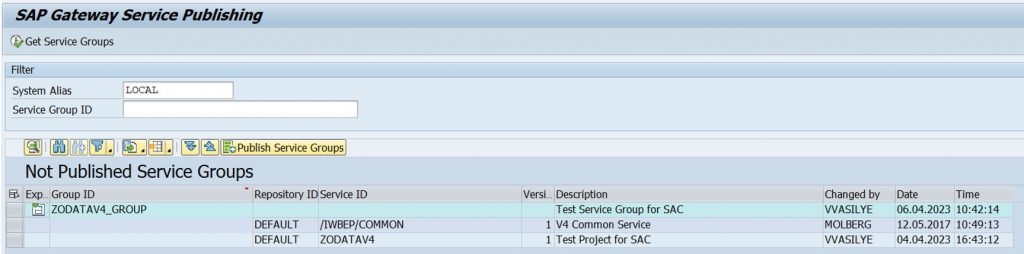
Highlight the desired group and click “Publish Service Groups”. Then return to the previous window. Now it shows the published group and the services assigned to it:
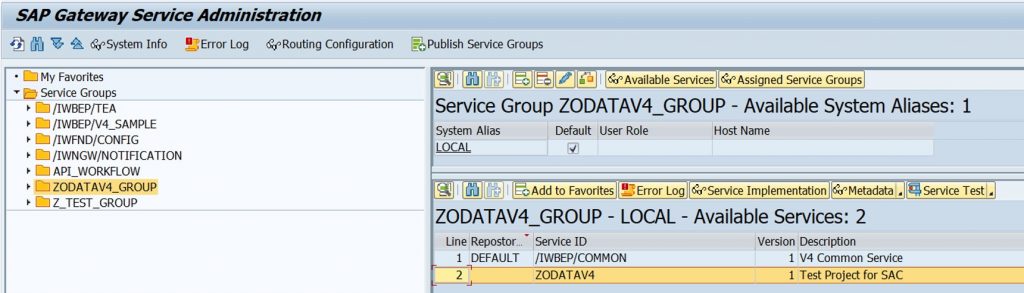
OData Service testing
The published service can be tested. This testing, if necessary, will allow you to fix the issues in the classes and methods described above by break-points enabling and switching to ABAP debugger. Testing can be started by highlighting our service as shown in the previous screenshot and selecting “Service Test -> Gateway Client”:

First of all, please, note the path displayed in the Request URI field:
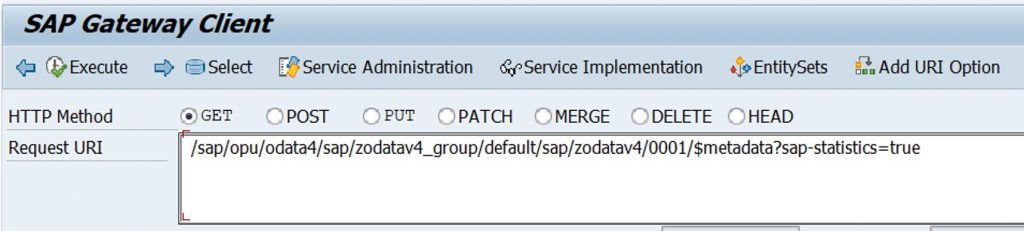
The starting part of this path upto the $ sign is the End-Point URL, which will be required later in the SAC settings. In our example, the End-Point URL turns out to be as follows: /sap/opu/odata4/sap/zodatav4_group/default/sap/zodatav4/0001/
To start testing, you must first switch the HTTP Method to POST. Secondly, in the Request URI, after the End-Point URL, you need to specify the name of the action that will be called. Thirdly, you need to pass the value of the input parameter to the action using JSON-Syntax. To test the service, a dummy chain ZPC_ODATA4_TEST was prepared. It simply calls a program that contains no code. Therefore, the launch of testing in the end looks like this:
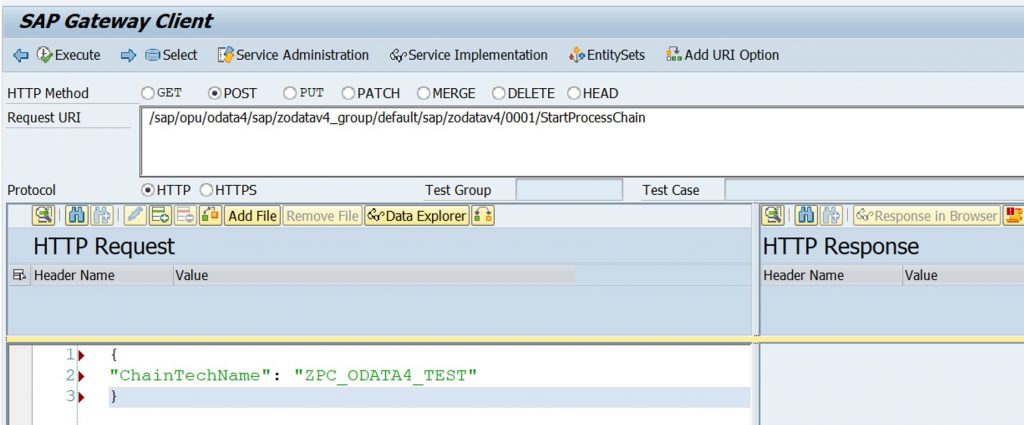
Now testing can be started by pressing the “Execute” button. The successful result looks like this:
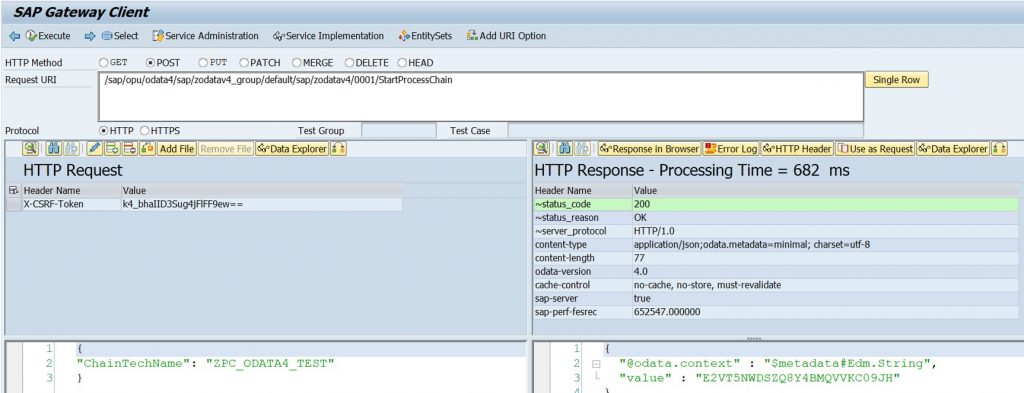
In the RSPC transaction we can verify that the service actually started the execution of the chain and returned the run ID:

OData Service utilization in SAC Analytic Application
Now we can use our OData Service in the SAC Analytic Application. For the demonstration, the simplest application was prepared. It contains three components: OData Service named ZODATAV4, button PC_START_BUTTON (labeled “Start Process Chain”), text field CHAIN_RUN_ID, designed to display the value of action return:
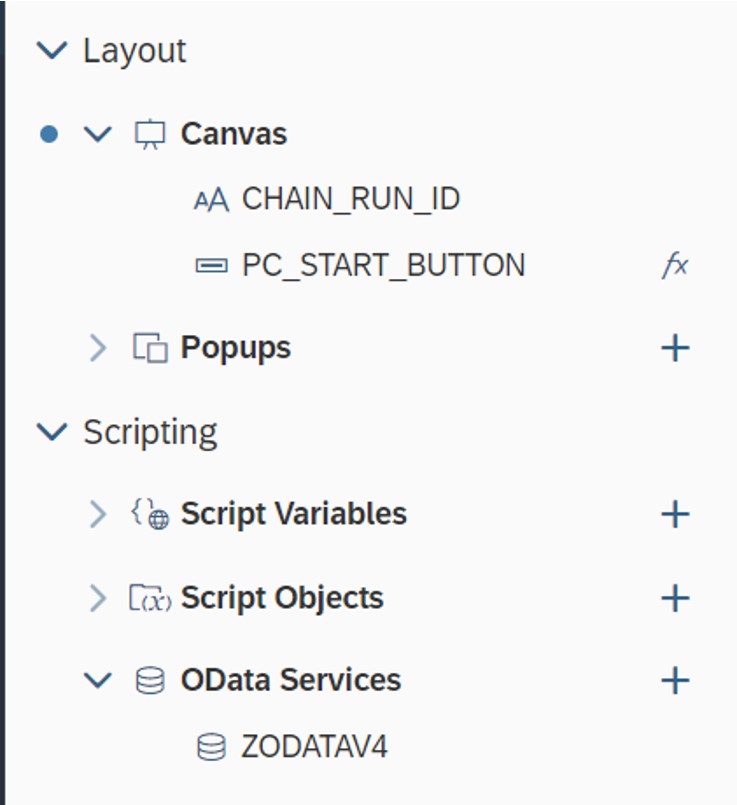
In the ZODATAV4 component settings you must specify the name of the Live Connection to that BW system where the service was created. See above for the required CORS settings for this system. You also need to specify the End-Point URL. The value of this parameter, corresponding to our example, was also indicated earlier:
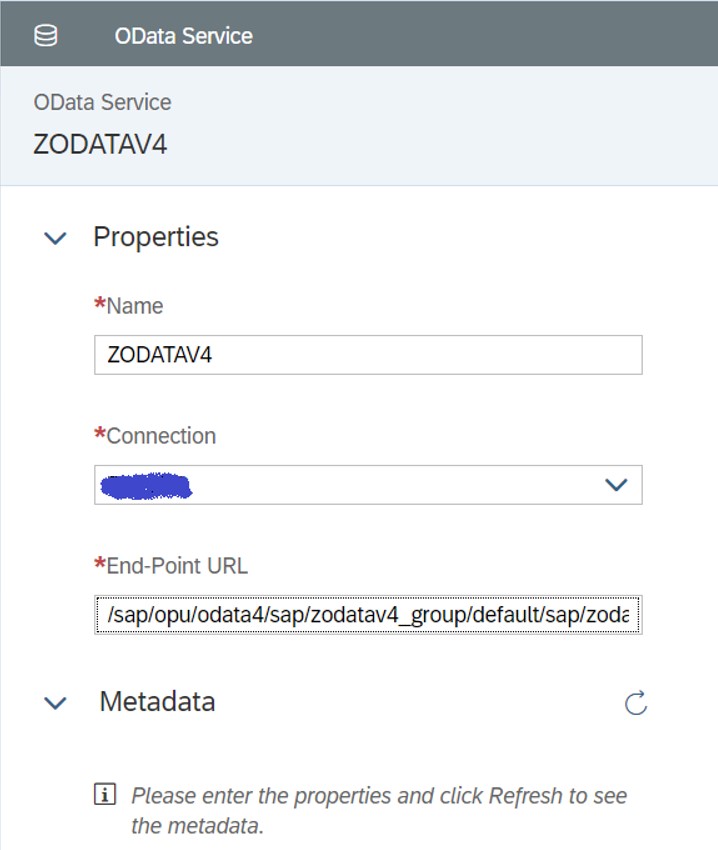
To check the connection to the Service, you need to click the “Refresh” button to the right of the Metadata section. If SSO between SAC and BW is not configured, then an additional window will appear at this moment asking for your BW credentials. If the connection to the Service is successful, then the Metadata section will display OData version used and a list of actions provided by this Service:
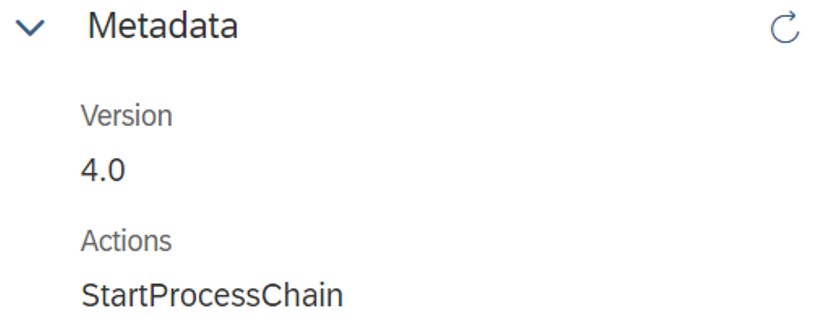
Now the action can be called with the onClick script for the PC_START_BUTTON button:
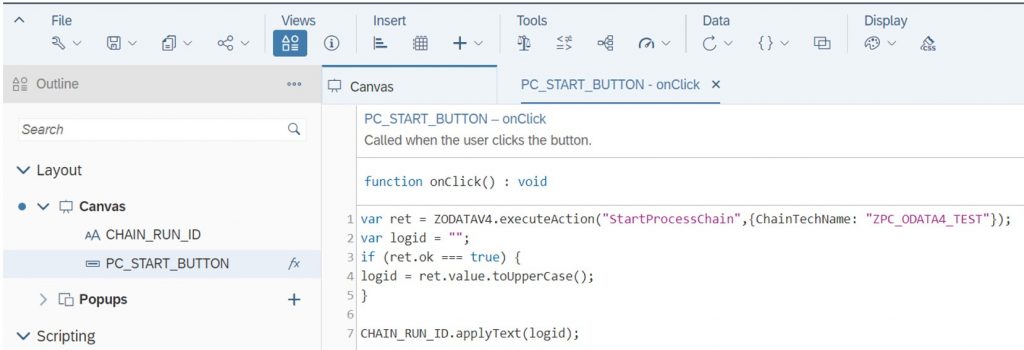
First line of the script shows how to call an action from the ZODATAV4 service connected to the SAC, how to specify the name of this action, how to pass the technical name of the chain to the input parameter of this action. Then, if there were no errors while calling the action, return value from the action is stored in the logid local variable. It, in turn, is displayed in the CHAIN_RUN_ID text field.
So, before the button is clicked, application window looks like this:
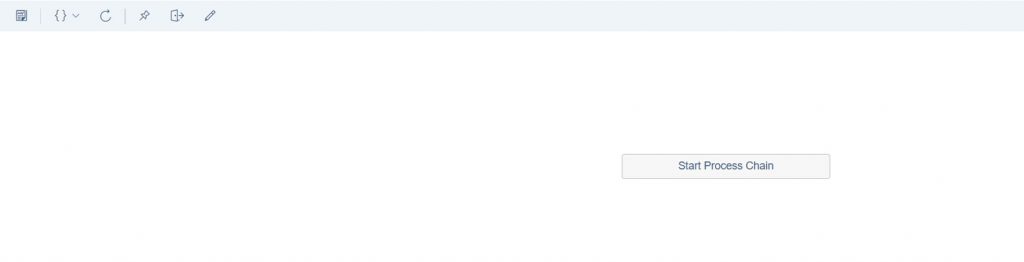
After clicking the button, chain run ID appears:
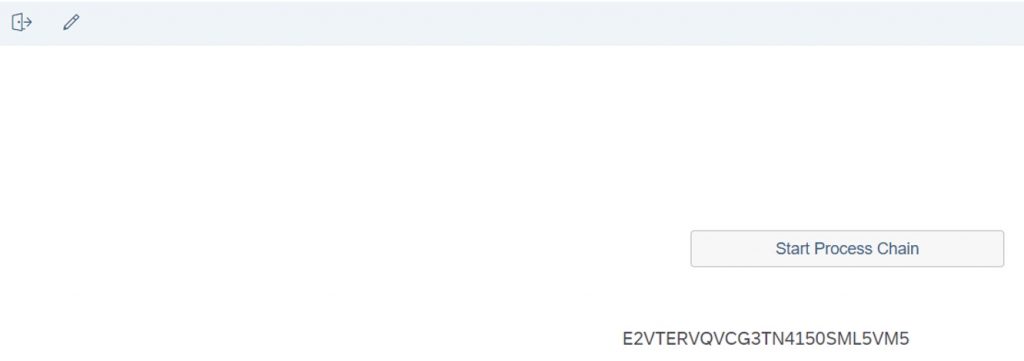
As before, we can check correctness of this number in the RSPC transaction.