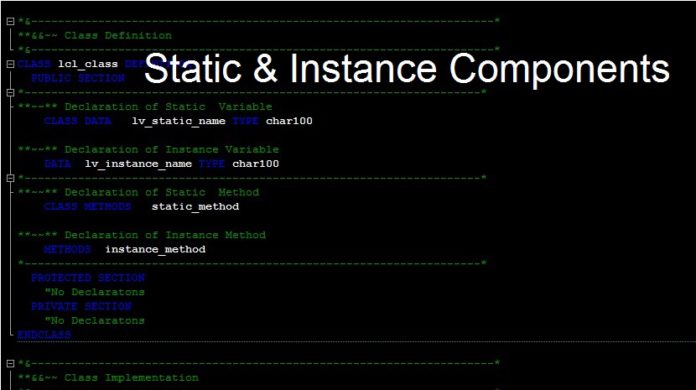
Static Attributes & Methods of the Class
In our Introduction to OOPs ABAP, we gave you the high-level understanding of the components used in ABAP Objects. Attributes, Methods, Events, Interfaces etc are the components of a class. We talked about them briefly in the last part. In this article, we would dive deeper into the Static Components and how to use the Static Components of the Class.
The static components of a class can be addressed using an Object TYPE REF and also by using the name of the class. This means they can be used independently of a class instance.
Static components are usually called by using “=>” Operator.
Declaring Static Attribute:
You need to declare the static Variables using CLASS-DATA Keyword.
- Static attributes are created once for the class.
- Memory is allocated for these attributes only once.
- We can change the value in memory.
CLASS-DATA : <Variable_Name> type <data_type>.
Declaring Static Method: For Static Method, you can have Importing / Exporting / Changing / Exception Parameters.
CLASS-METHODS: <Method_name>.
( OR )
CLASS-METHODS : <method_name> IMPORTING a type i
EXPORTING b type i
CHANGING c type i
EXCEPTIONS e .
Note: Instance Attributes/Variables are declared using DATA and Instance Methods are declared using METHODS.
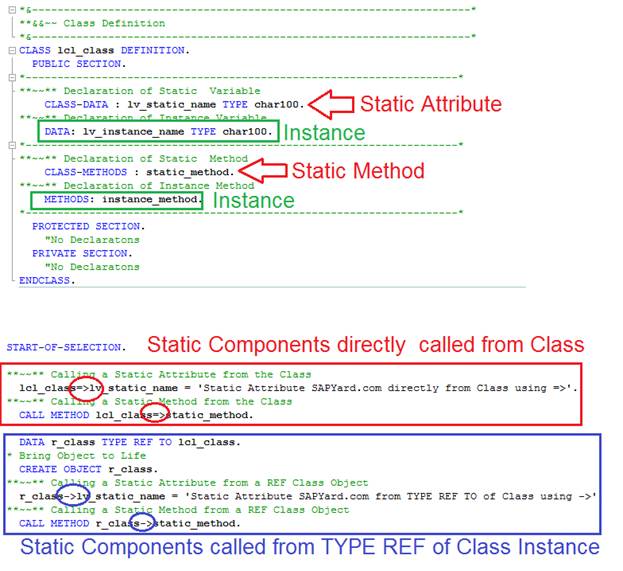
If you understand the above figure, you are good with this article. No need to read further. Watch some Soccer, American Football or IPL now.
If you already understand and still want to know the nuisances of Static Components, keep reading.
Case 1 – Accessing Static Method & Static Variable (Attribute) from Class Name directly
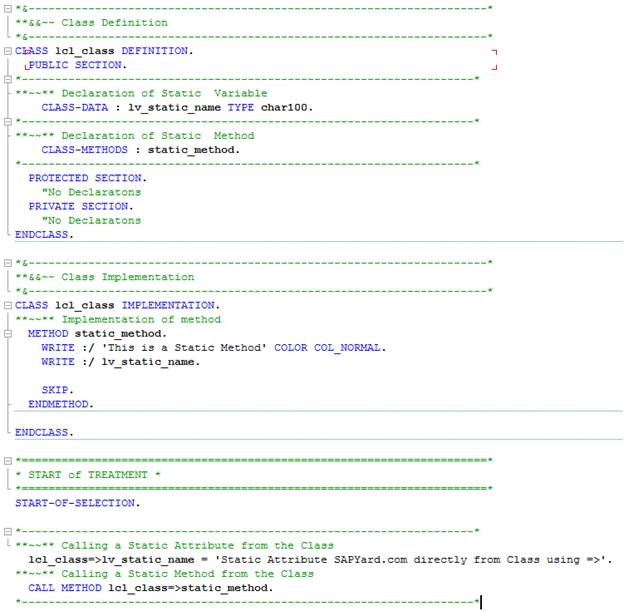
This is a straight case. The class lc_class calls the static method (static_method defined using CLASS-METHODS) using the operator “=>”. The method, in turn, used static variable lv_static_name which is defined using CLASS-DATA.
Output
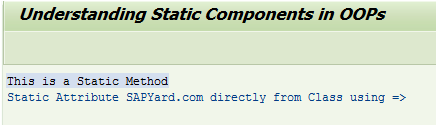
Case 2 – Accessing Static Method & Static Variable (Attribute) using TYPE REF of Class
As mentioned above, the static method can be accessed directly by the Object (class) or it’s reference (TYPE REF of). The only difference it, instead of “=>” we need to use “->” to access the components.
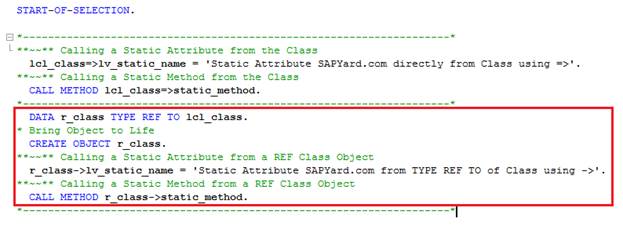
Output
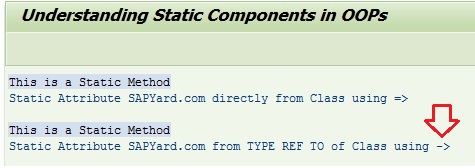
Case 3 – Accessing Instance Attribute (Variable) in Static Method.
Let us define a non-static attribute lv_instance_name.
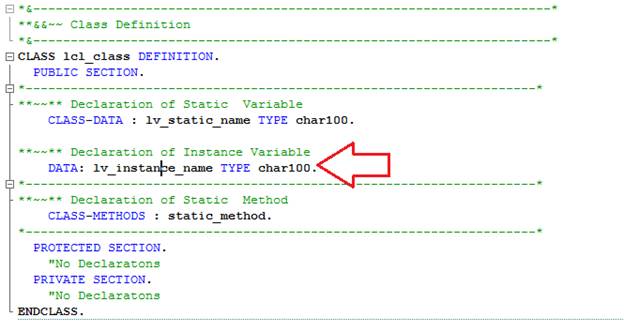
Let us try to use the non-static attribute in the static method as shown below.
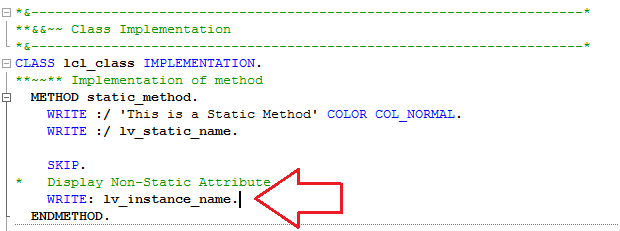
Try to activate the code. OOPs, you get an error. Looks like, Static Methods can access only Static Attributes.

Since we cannot access the instance attribute in Static Method, let us try to access Static Data in Instance Method.
Case 4 – Accessing Static Attribute (Variable) in Instance Method.
Define an instance method and implement it as shown below.
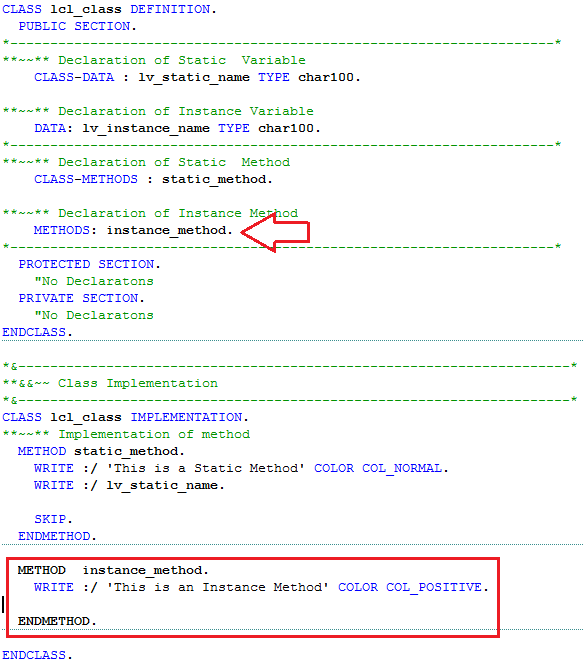
Now let us try to access the static attribute of the same class in the instance method.

When you activate the code, it works. Yes, static attributes can be accessed from all methods (static and instance).
So, you now know another difference between Static and Instance Attributes.
Case 5 – Calling Instance Method.
Let us access both static and instance attributes in instance method as shown below.
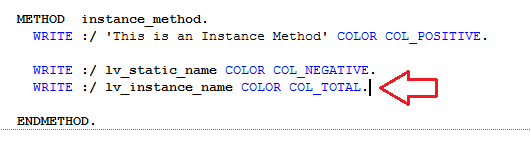
Let us try to call the instance method using “=>” operator directly from the class name.
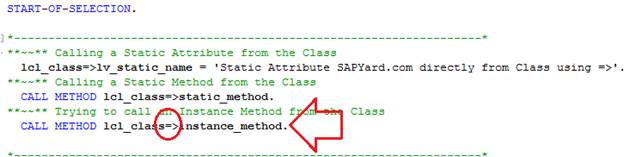
Not surprising. We get an error. “=>” is only for Static Components.

Let us try to access instance method using ‘->’ operator from the class name.
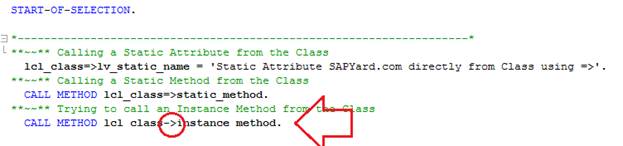
It is forbidden too. You cannot use “->’ directly from the class name.

The right way to access instance method is from the TYPE REF of the class object.
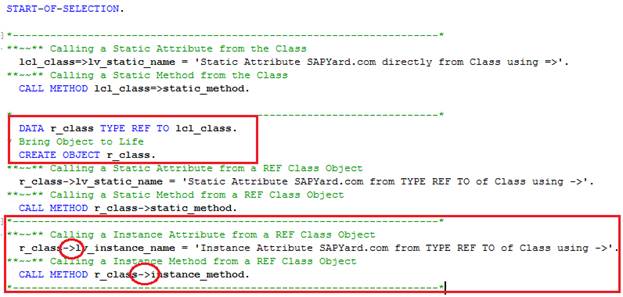
Let us check the final output.
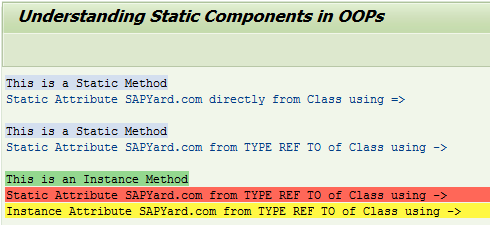
3 POINTS TO TAKE HOME FROM THIS TUTORIAL
- Static attributes/variables are like global variables for function module, and it can be accessed by any methods (static or instance) of that class.
- Instance attributes/variables cannot be used in Static Methods.
- Static Methods cannot be re-defined. OO Programming without Polymorphism is of not much use. Static methods are operable independently. Static Methods are directly called at the Class Implementation, so they are not tied to any reference instance class. So, the static methods cannot be inherited and redefined.
WHY SHOULD WE OPT FOR INSTANCE METHOD INSTEAD OF STATIC?
Using Static Method looks simpler than Instance Method (as classname=>static_method is easier than TYPE REF of and CREATE Object and then r_object->instance_method i.e. 3 steps – declaring, instantiating and calling), but since Static Methods cannot be re-defined, that should be one big deterrent to use it. Between Static and Instance Methods, always go for Instance method in your design.
If you are designing a utility class (not helper class), then you can use static components whose value needs to be static. Please understand, if the values of static attributes are set, it won’t be cleared till the session is complete. In other words, static attributes are bound to the memory for the entire session.
The complete code used for this article is below.
*&---------------------------------------------------------------------*
*& Report YRAM_OOABAP2
*======================================================================*
* Project : SAP Object Oriented ABAP *
* Description : Demonstrating about the Static Components *
*======================================================================*
REPORT yram_ooabap2.
*&---------------------------------------------------------------------*
**&&~~ Class Definition
*&---------------------------------------------------------------------*
CLASS lcl_class DEFINITION.
PUBLIC SECTION.
*--------------------------------------------------------------------*
**~~** Declaration of Static Variable
CLASS-DATA : lv_static_name TYPE char100.
**~~** Declaration of Instance Variable
DATA: lv_instance_name TYPE char100.
*--------------------------------------------------------------------*
**~~** Declaration of Static Method
CLASS-METHODS : static_method.
**~~** Declaration of Instance Method
METHODS: instance_method.
*--------------------------------------------------------------------*
PROTECTED SECTION.
"No Declaratons
PRIVATE SECTION.
"No Declaratons
ENDCLASS.
*&---------------------------------------------------------------------*
**&&~~ Class Implementation
*&---------------------------------------------------------------------*
CLASS lcl_class IMPLEMENTATION.
**~~** Implementation of method
METHOD static_method.
WRITE :/ 'This is a Static Method' COLOR COL_NORMAL.
WRITE :/ lv_static_name.
SKIP.
ENDMETHOD.
METHOD instance_method.
WRITE :/ 'This is an Instance Method' COLOR COL_POSITIVE.
WRITE :/ lv_static_name COLOR COL_NEGATIVE.
WRITE :/ lv_instance_name COLOR COL_TOTAL.
ENDMETHOD.
ENDCLASS.
*======================================================================*
* START of TREATMENT *
*======================================================================*
START-OF-SELECTION.
*--------------------------------------------------------------------*
**~~** Calling a Static Attribute from the Class
lcl_class=>lv_static_name = 'Static Attribute SAPSPOT.com directly from Class using =>'.
**~~** Calling a Static Method from the Class
CALL METHOD lcl_class=>static_method.
*--------------------------------------------------------------------*
DATA r_class TYPE REF TO lcl_class.
* Bring Object to Life
CREATE OBJECT r_class.
**~~** Calling a Static Attribute from a REF Class Object
r_class->lv_static_name = 'Static Attribute SAPSPOT.com from TYPE REF TO of Class using ->'.
**~~** Calling a Static Method from a REF Class Object
CALL METHOD r_class->static_method.
*--------------------------------------------------------------------*
**~~** Calling a Instance Attribute from a REF Class Object
r_class->lv_instance_name = 'Instance Attribute SAPSPOT.com from TYPE REF TO of Class using ->'.
**~~** Calling a Instance Method from a REF Class Object
CALL METHOD r_class->instance_method.
*--------------------------------------------------------------------*